Backend Integration of SSO
If you are doing an entirely backend integration with Stytch (using our Direct APIs, or the Backend SDKs) the below sequence outlines how Single Sign-On (SSO) authentication will work:
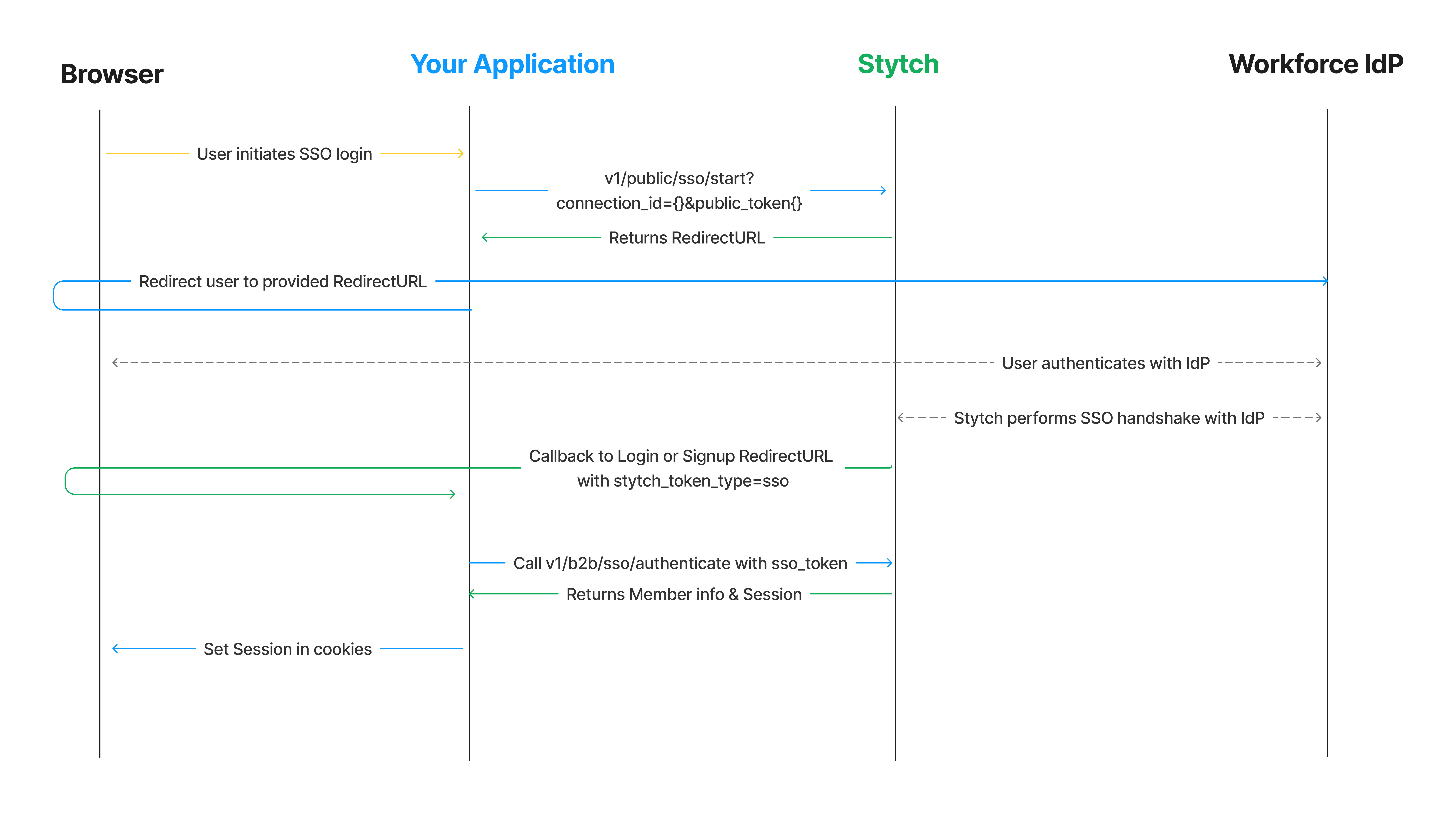
Complete config steps
If you haven't done so already complete the steps in the SSO Integration Guide Start Here
2Configure callback
Stytch will make a callback to the Login or Signup RedirectURL that you specified in the Stytch dashboard. Your application should handle checking the stytch_token_type for the callback, and call the appropriate authenticate() method to finish the login process.
If your RedirectURL was http://localhost:3000/authenticate you would add the following route to your application:
@app.route("/authenticate", methods=["GET"])
def authenticate() -> str:
token_type = request.args["stytch_token_type"]
if token_type == "sso":
resp = stytch_client.sso.authenticate(sso_token=request.arg["token"])
if resp.status_code != 200:
return "something went wrong authenticating token"
else:
## handle other authentication method callbacks
return "unsupported auth method"
## member is successfully logged in
member = resp.member
session["stytch_session"] = resp.session_jwt
return member.json()
3Initiate SSO
Now that your application is ready to handle the authentication callback, you can test out an end-to-end authentication flow!
Using the ConnectionID for the SSO Connection you created earlier, and your Public Token (found in the Stytch Dashboard under API Keys) enter the following into your browser:
https://test.stytch.com/v1/public/sso/start?connection_id={connection_id}&public_token={public_token}
This will automatically redirect you to the workforce IdP that the SSO Connection is tied to, and if you’re not already logged in you will be prompted to authenticate on the IdP.
4(Optional) Build frontend for selecting SSO connection
In a real application, you’ll need a UI that will allow the end user to identify the Organization they wish to log into – and select the SSO Connection to use if there are multiple options.
We recommend using Organization-specific login pages so that end users can easily navigate to their Organization’s instance to login (e.g. your-app.com/<organization_slug> or <organization_slug>.your-app.com), and be shown the SSO Connection(s) for that Organization. We do not recommend surfacing the SSO Connection(s) based on the end user's email domain, as the assumption that 1 email domain maps to 1 Organization breaks at scale.
Once the Organization has been identified, you can call GetOrganization and use the information in the response to display the active SSO Connections for the Organization to the end user to select from, or use the DefaultConnectionID if there is only one valid SSO Connection for the Organization.
@app.route("/org/<string:slug>", methods=["GET"])
def org_index(slug: str):
# Check for active member session, if present show logged in view
# Otherwise show login screen
resp = stytch_client.organizations.search(query=SearchQuery(operator="AND", operands=[{
"filter_name": "organization_slugs",
"filter_value": [slug]}
]))
if resp.status_code != 200 or len(resp.organizations) == 0:
return "Error fetching org"
organization = resp.organizations[0]
return render_template(
'organizationLogin.html',
public_token=STYTCH_PUBLIC_TOKEN,
org_name=organization.organization_name,
sso_connections=organization.sso_active_connections
)