Google One Tap
Google One Tap is a popular, low-friction authentication method that enables end users to select the Google account they wish to use to sign in with a single tap.
The Google One Tap prompt will appear in the top right of the end user's browser, and will look something like this:
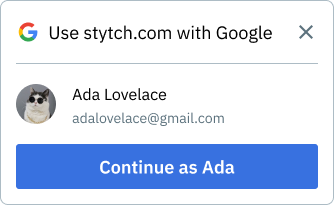
Summary of Key Differences
For a quick overview of the differences between Google OAuth and Google One Tap, see the table below:
Standard Google OAuth | Google One Tap | |
---|---|---|
Issued OAuth Tokens | access_token and refresh_token | None (use standard OAuth if tokens are needed) |
Available Stytch Integration Methods | Backend, Headless Frontend, Pre-built UI | Headless Frontend, Pre-built UI |
Supported Browsers | All | Not supported in Arc or Brave; Blocked by certain browser extensions |
Headless SDK Reference Docs | Discovery Sign-up and Login and Organization Login | Discovery Sign-up and Login and Organization Login |
Adding One Tap to your Google OAuth integration
If you don't already have Google OAuth configured in the Stytch dashboard, follow the steps here.
Make sure to follow the setup instructions for adding your application's URI as an Authorized Javascript Origin in your Google OAuth credential. For example, if you plan on showing One Tap at https://example.com/login, enter https://example.com. If you're using localhost for testing, we recommend adding both http://localhost and http://locahost:[port].
Headless frontend integration
If you don't already have Google OAuth set up in the headless SDK, follow the steps here.
Discovery sign-up or login
If you wish to use Google One Tap for the Discovery flow, you can call the oauth.googleOneTap.discovery.start() method to display the Google One Tap prompt in the upper right of the user's browser.
If the end user clicks on the prompt to sign in with Google One Tap, Stytch will verify the response and redirect to the Discovery RedirectURL you specified in the Stytch dashboard earlier.
The code snippets below show how to configure the One Tap prompt to appear when prompted by a user action (i.e Button click), but you could also configure the prompt to appear on page load/render.
In our React SDK, it might look something like this:
import { useStytchB2BClient } from '@stytch/react/b2b';
export const GoogleOneTapDiscovery = () => {
const stytchClient = useStytchB2BClient();
const showGoogleOneTapDiscovery = () =>
stytchClient.oauth.googleOneTap.discovery.start();
return <button onClick={showGoogleOneTapDiscovery}>Show Google One Tap Discovery</button>;
};
In our vanilla JS SDK, you would add:
<script>
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('PUBLIC_TOKEN');
document.getElementById('show-google-one-tap-discovery').onclick = () => stytch.oauth.googleOneTap.discovery.start({
discovery_redirect_url: 'https://example.com/authenticate',
});
</script>
<button id="show-google-one-tap-discovery">Show Google One Tap Discovery</button>
Organization login
If you wish to use Google One Tap, you can call the oauth.googleOneTap.start() method to display the Google One Tap prompt in the upper right of the user's browser.
If the end user clicks on the prompt to sign in with Google One Tap, Stytch will verify the response and redirect to the Login or Signup RedirectURL you specified in the Stytch dashboard earlier.
The code snippets below show how to configure the One Tap prompt to appear when prompted by a user action (i.e Button click), but you could also configure the prompt to appear on page load/render.
In our React SDK, it might look something like this:
import { useStytchB2BClient } from '@stytch/react/b2b';
export const GoogleOneTap = () => {
const stytchClient = useStytchB2BClient();
const showGoogleOneTap = () =>
stytchClient.oauth.googleOneTap.start({
organization_id: 'organization-test-07971b06-ac8b-4cdb-9c15-63b17e653931',
});
return <button onClick={showGoogleOneTap}>Show Google One Tap</button>;
};
In our vanilla JS SDK, you would add:
<script>
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('PUBLIC_TOKEN');
document.getElementById('show-google-one-tap').onclick = () => stytch.oauth.googleOneTap.start({
organization_id: 'organization-test-07971b06-ac8b-4cdb-9c15-63b17e653931',
});
</script>
<button id="show-google-one-tap">Show Google One Tap</button>
Pre-built UI frontend integration
If you don't already have Google OAuth set up in the pre-built UI components, follow the steps here.
To add One Tap, you can add the one_tap field to your providers list in your OAuth UI configuration.
If the only product specified is oauth, and the only OAuth provider specified is google with one_tap set to true, the login form will not be displayed. Instead, we will only show the Google one tap prompt in the upper right of the user's browser.
Google One Tap is not supported on all browsers, and can sometimes be blocked by certain browser extensions. If Google OAuth is your only login method, we recommend only utilizing One Tap in targeted locations, and not on your core login page
import React from 'react';
import { StytchB2B } from '@stytch/react/b2b';
const Login = () => {
// Example config for the Discovery auth flow that only shows the One Tap prompt
var oneTapOnlyDiscoveryConfig = {
authFlowType: "Discovery",
products: ['oauth'],
oauthOptions: {
providers: [{ type: 'google', one_tap: true }]
},
};
// Example config for the Organization auth flow
// This will show a Google OAuth button, a Microsoft OAuth button, and the Google One Tap prompt
var oneTapOrganizationConfig = {
authFlowType: "Organization",
products: ['oauth'],
oauthOptions: {
providers: [{ type: 'google', one_tap: true }, { type: 'microsoft' }]
},
};
return (
<div>
// Choose either of the config options
<StytchB2B config={oneTapOrganizationConfig} />
</div>
);
};
export default Login;
<!DOCTYPE html>
<html lang="en">
<body>
<div class="app">
<div>
<div id="stytch-sdk"></div>
</div>
</div>
<script>
import { StytchB2BUIClient } from '@stytch/vanilla-js/b2b';
const stytch = new StytchB2BUIClient('PUBLIC_TOKEN');
// Example config for the Discovery auth flow that only shows the One Tap prompt
var oneTapOnlyDiscoveryConfig = {
authFlowType: "Discovery",
products: ['oauth'],
oauthOptions: {
providers: [{ type: 'google', one_tap: true }]
},
};
// Example config for the Organization auth flow
// This will show a Google OAuth button, a Microsoft OAuth button, and the Google One Tap prompt
var oneTapOrganizationConfig = {
authFlowType: "Organization",
products: ['oauth'],
oauthOptions: {
providers: [{ type: 'google', one_tap: true }, { type: 'microsoft' }]
},
};
stytch.mount({
elementId: "#stytch-sdk",
// Choose either of the config options
config: oneTapOrganizationConfig,
});
</script>
</body>
</html>