Headless Frontend Integration of Email Magic Links
In Stytch’s B2B product there are two different versions of the EML authentication flow:
- Discovery Authentication: used for self-serve Organization creation or login prior to knowing the Organization context
- Organization-specific Authentication: used when you already know the Organization that the end user is trying to log into
The guides below cover how to offer Email Magic Links for both scenarios, using Stytch's headless frontend SDK.
Discovery Sign-Up or Login
The discovery flow is designed for situations where your end users are signing up or logging in from a central landing page, and have not specified which organization they are trying to access or are attempting to create a new Organization.
The sequence for how this flow works when using a headless integration approach is as follows:
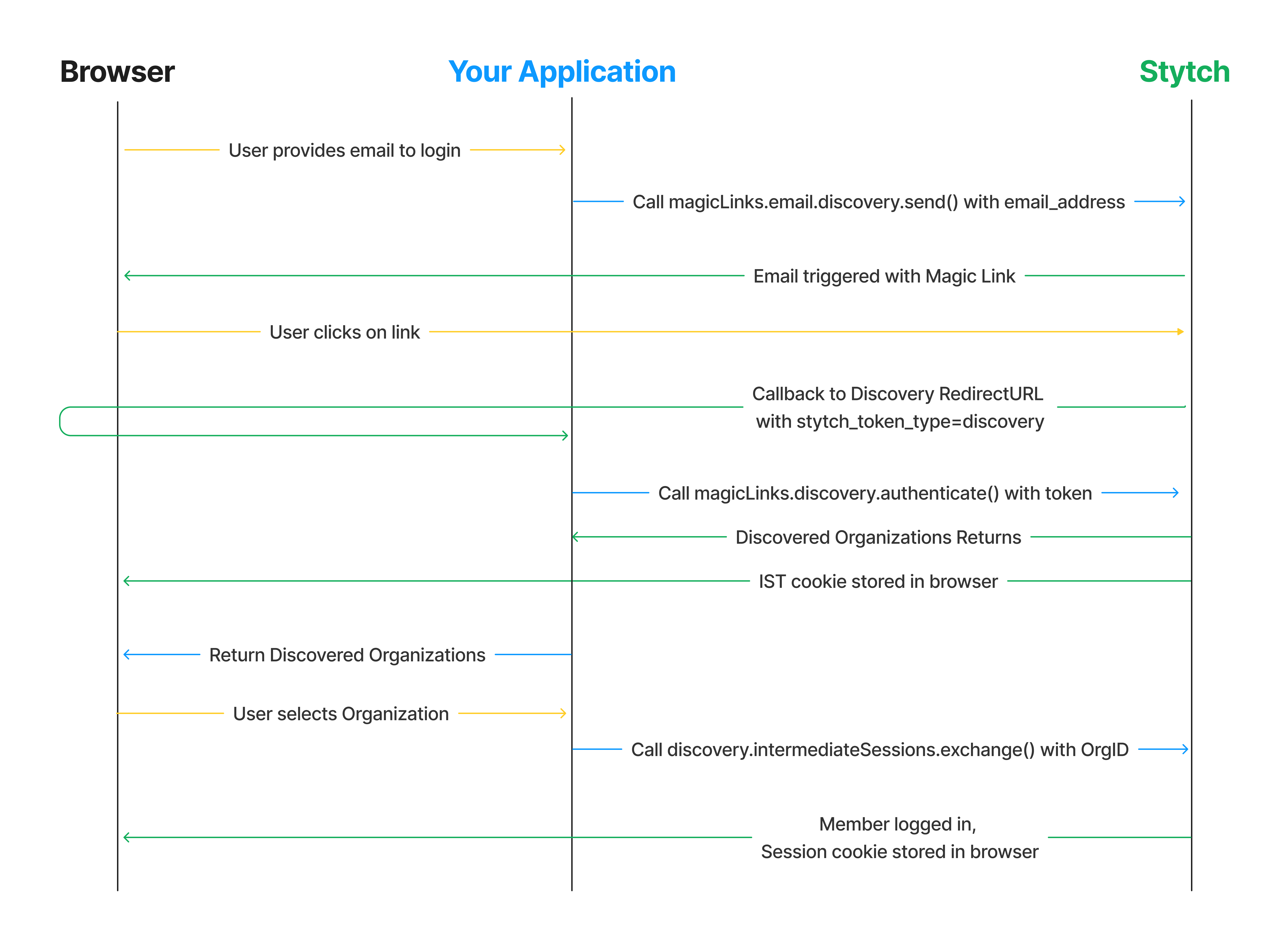
1Complete config steps
If you haven't done so already:
- Complete the steps in the EML Integration Guide Start Here
- Enable the Frontend SDK in your Stytch Dashboard
- In the Frontend SDK config, enable Create Organizations under "Enabled Methods"
2Initiate discovery flow from login page
In your application’s UI, introduce a way for end users to input their email and trigger a magicLinks.email.discovery.send() method call with the provided email.
For example, with our React SDK:
import { useStytchB2BClient } from '@stytch/react/b2b';
export const Login = () => {
const stytch = useStytchB2BClient();
const sendDiscoveryEmail = () => {
stytch.magicLinks.email.discovery.send({
email_address: 'sandbox@stytch.com',
});
};
return <button onClick={sendDiscoveryEmail}>Send email</button>;
};
Using the vanilla JS SDK this would look as follows:
<button onclick="sendDiscoveryEmail()">Send email</button>
<script>
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('STYTCH_PUBLIC_TOKEN');
const sendDiscoveryEmail = () => {
stytch.magicLinks.email.discovery.send({
email_address: 'sandbox@stytch.com',
});
};
</script>
3Configure redirect
Stytch will redirect the user to the Discovery RedirectURL you specified on the Redirect URLs page earlier. Upon receiving this callback you will exchange the token in the call for a list of Discovered Organizations that the user can choose to log into. An intermediate_session_token (IST) will also be returned, but the headless SDK will take care of setting this in cookies and including it in the follow up calls.
In React this looks like:
export const DiscoveryAuthenticate = () => {
const stytch = useStytchB2BClient();
useEffect(() => {
const authenticate = async () => {
const token = new URLSearchParams(window.location.search).get('token');
const tokenType = new URLSearchParams(window.location.search).get('stytch_token_type');
if (token && tokenType === 'discovery') {
const { email_address, discovered_organizations } =
await stytch.magicLinks.discovery.authenticate({
discovery_magic_links_token: token,
});
// surface discovered organizations to user to select from
console.log({ email_address, discovered_organizations });
}
};
authenticate();
}, [stytch.magicLinks.discovery]);
return <div>Loading</div>;
};
In vanilla Javascript this looks like:
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('STYTCH_PUBLIC_TOKEN');
const token = new URLSearchParams(window.location.search).get('token');
const tokenType = new URLSearchParams(window.location.search).get('stytch_token_type');
if (token && tokenType === 'discovery') {
const { email_address, discovered_organizations } = stytch.magicLinks.discovery.authenticate({
discovery_magic_links_token: token
});
// surface discovered organizations to user to select from
console.log({ email_address, discovered_organizations });
}
4Handle user selection
If the end user selects to login to an existing Organization, you can call the exchange intermediate session method with the selected OrgID.
For example, in React you would add:
import React, { useEffect } from 'react';
import { useStytchB2BClient } from '@stytch/react/b2b';
export const ExchangeIntermediateSession = () => {
const stytch = useStytchB2BClient();
useEffect(() => {
stytch.discovery.intermediateSessions.exchange({
organization_id: 'organization-test-07971b06-ac8b-4cdb-9c15-63b17e653931'
});
});
return <div>Log In</div>;
};
In vanilla Javascript you would add the following:
<button onclick="exchangeIntermediateSession()">Log In</button>
<script>
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('STYTCH_PUBLIC_TOKEN');
const ExchangeIntermediateSession = () => {
stytch.discovery.intermediateSessions.exchange({
organization_id: 'organization-test-07971b06-ac8b-4cdb-9c15-63b17e653931'
});
};
</script>
You can also optionally allow users the ability to create a new Organization instead of logging into an existing one. In order to enable this, ensure that you have enabled Create Organizations in the Frontend SDK page of the Stytch Dashboard.
To support creating a new Organization through discovery in your application, add the following code with our React SDK:
import React, { useEffect } from 'react';
import { useStytchB2BClient } from '@stytch/react/b2b';
export const CreateOrganization = () => {
const stytch = useStytchB2BClient();
useEffect(() => {
stytch.discovery.organizations.create();
});
return <div>Create Organization</div>;
};
Or with our vanilla Javascript SDK:
<button onclick="createOrganization()">Create Organization</button>
<script>
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('STYTCH_PUBLIC_TOKEN');
const createOrganization = () => {
stytch.discovery.organizations.create();
};
</script>
The discovery.organizations.create() method has two optional parameters: organization_slug and organization_name where you can pass custom values. If organization name and slug are not provided Stytch will auto generate a name and slug based on the end user’s email address.
5Test it out
Run your application and test out doing discovery login and sign-up!
Organization Login
If end users of your application login via a page that indicates which Organization they are trying to log into (e.g. <org-slug>.your-app.com or your-app.com/team/<org-slug>) you can offer organization login on that page.
The high level flow using our headless SDK is as follows:
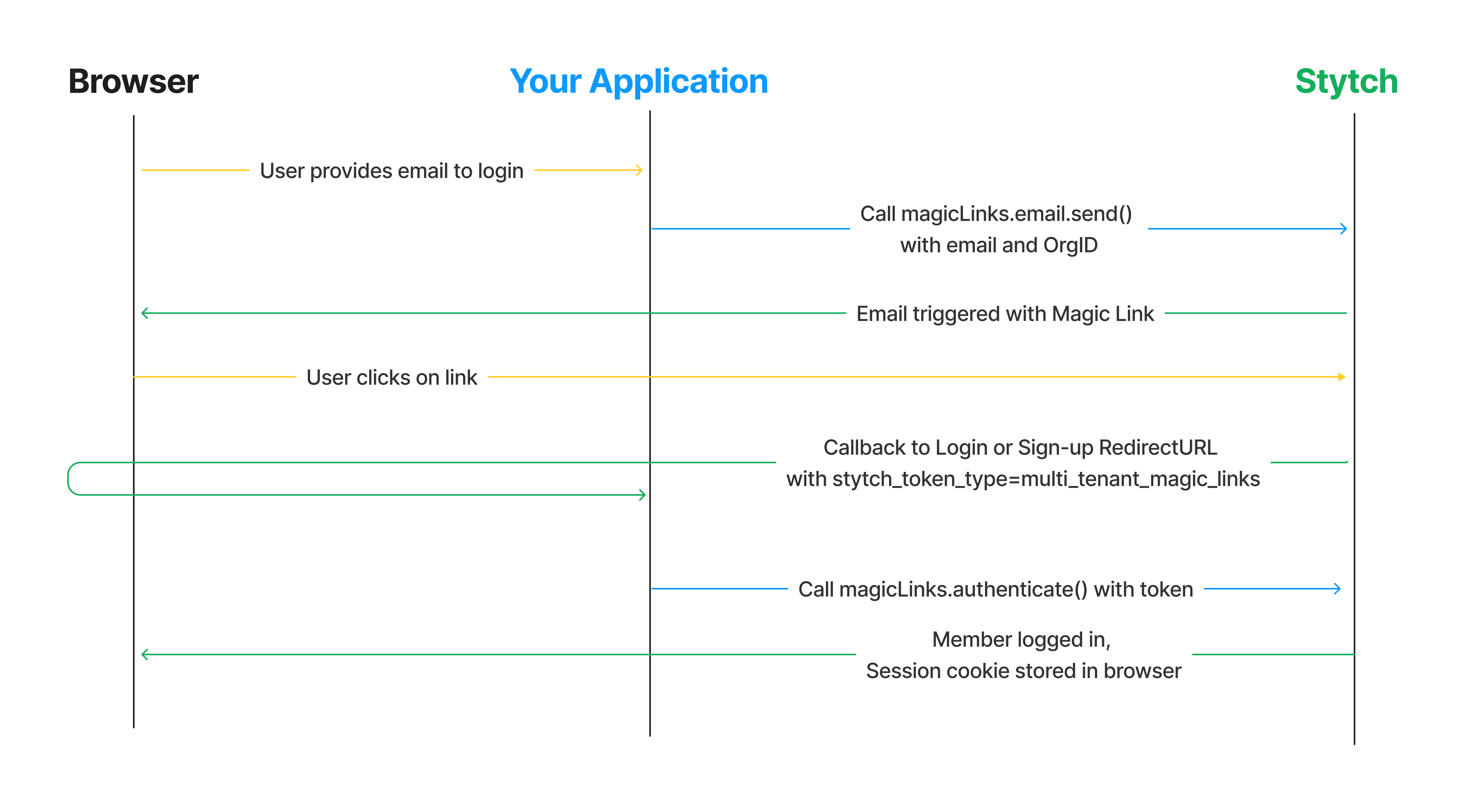
1Complete config steps
If you haven't done so already:
- Complete the steps in the EML Integration Guide Start Here, including creating an Organization
- Enable the Frontend SDKs in your Stytch Dashboard
2Support email input on organization login page
On your login page for the organization, take in a user's email address and call the magicLinks.email.loginOrSignup() method.
In our React SDK this would look like:
import { useStytchB2BClient } from '@stytch/react/b2b';
export const Login = () => {
const stytch = useStytchB2BClient();
const sendEmailMagicLink = () => {
stytch.magicLinks.email.loginOrSignup({
email_address: 'sandbox@stytch.com',
organization_id: 'organization-test-07971b06-ac8b-4cdb-9c15-63b17e653931',
});
};
return <button onClick={sendEmailMagicLink}>Send email</button>;
};
In vanilla Javascript this would look as follows:
<button onclick="sendEmailMagicLink()">Login with email</button>
<script>
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('STYTCH_PUBLIC_TOKEN');
const sendEmailMagicLink = () => {
stytch.magicLinks.email.loginOrSignup({
email_address: 'sandbox@stytch.com',
organization_id:'organization-test-07971b06-ac8b-4cdb-9c15-63b17e653931',
});
};
</script>
3Configure callback
Stytch will redirect the user to the Login or Signup RedirectURL that you specified in the magicLinks.email.loginOrSignup() call, or the relevant default in the Redirect URLs page of the Stytch Dashboard. The URL’s query parameters will contain stytch_token_type=multi_tenant_magic_links and an authentication token. Your application should extract the token from the URL and call the appropriate authentication method to finish the login process.
In our React SDK you would add the following:
import React, { useEffect } from 'react';
import { useStytchB2BClient, useStytchMemberSession } from '@stytch/react/b2b';
export const Authenticate = () => {
const stytch = useStytchB2BClient();
const { session } = useStytchMemberSession();
useEffect(() => {
if (session) {
window.location.href = 'https://example.com/profile';
} else {
const token = new URLSearchParams(window.location.search).get('token');
stytch.magicLinks.authenticate({
magic_links_token: token,
session_duration_minutes: 60,
});
}
}, [stytch, session]);
return <div>Loading</div>;
};
In vanilla Javascript you would add:
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('STYTCH_PUBLIC_TOKEN');
const token = new URLSearchParams(window.location.search).get('token');
stytch.magicLinks.authenticate({
magic_links_token: token,
session_duration_minutes: 60,
});
3Test it out
Run your application and test out the flow using the Organization that you created earlier!