Headless Frontend Integration
If you are using Stytch's headless JS SDK to integrate Single Sign-On (SSO), the sequence flow is as follows:
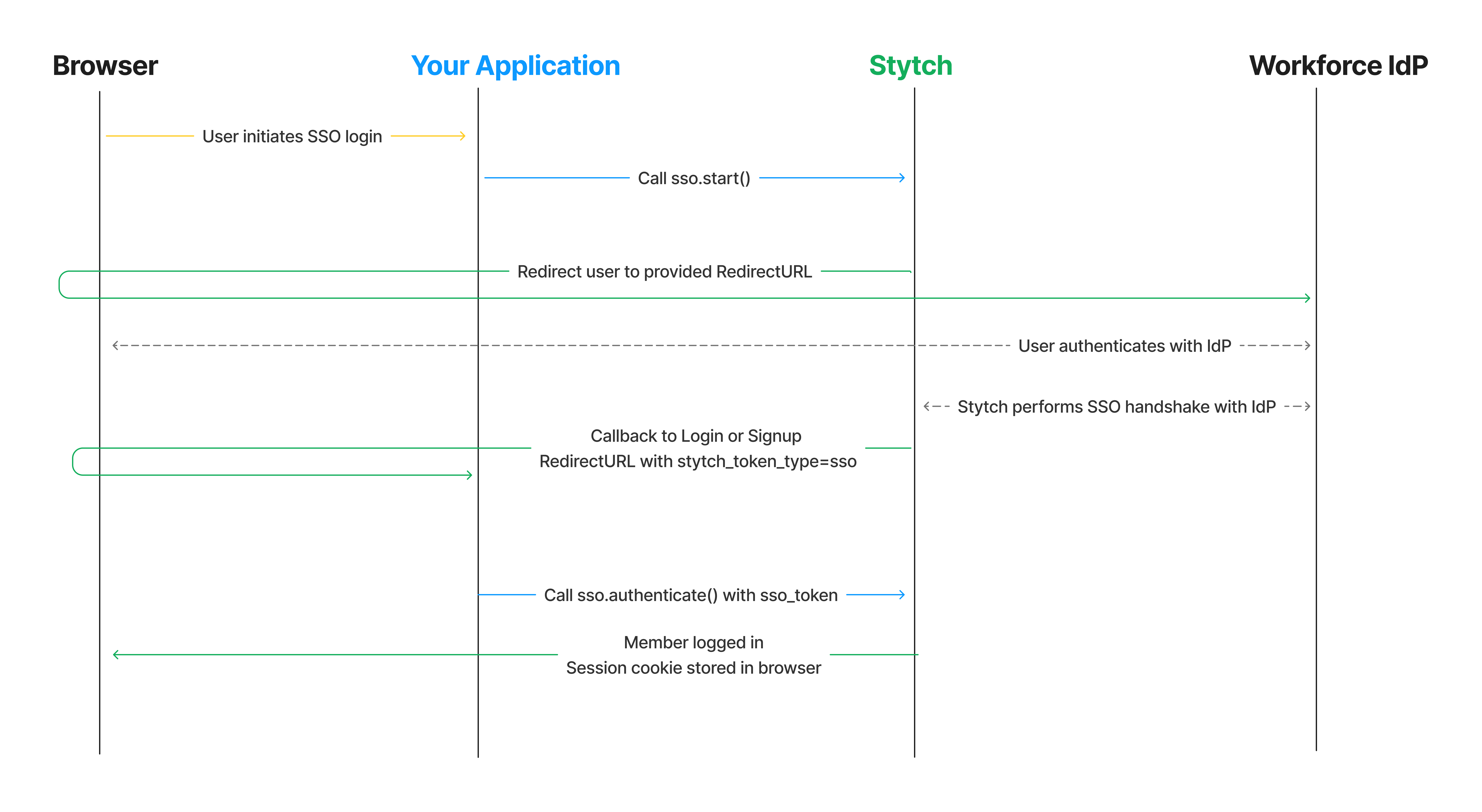
Complete config steps
If you haven't done so already:
- Complete the steps in the SSO Integration Guide Start Here
- Enable the Frontend SDKs in your Stytch Dashboard
2Configure login start
In your application’s UI, use the Stytch client’s sso.start method to start SSO. Using our React SDK this looks like:
import { useStytchB2BClient } from '@stytch/react/b2b';
export const Login = ({ organization }) => {
const stytchClient = useStytchB2BClient();
const startSSO = (connection_id) =>
stytchClient.sso.start({
connection_id: connection_id,
});
return <button onClick={startSSO(organization.default_connection_id)}>Log in with SSO </button>;
};
Using the vanilla JS in HTML, this looks like:
<script>
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('PUBLIC_TOKEN');
document.getElementById('login-with-sso').onclick = () => stytch.sso.start({
connection_id: 'saml-connection-test-51861cbc-d3b9-428b-9761-227f5fb12be9',
});
</script>
<button id="login-with-sso">Login with SSO</button>
3Configure callback
Stytch will redirect the user to the Login or Signup RedirectURL that you specified in the sso.start call, or the default specified in the Stytch dashboard. The URL’s query parameters will contain stytch_token_type=sso and token containing an authentication token. Your application should extract the token from the URL and call the appropriate authentication method to finish the login process.
Using our React SDK this will look like:
import React, { useEffect } from 'react';
import { useStytchB2BClient, useStytchMemberSession } from '@stytch/react/b2b';
export const Authenticate = () => {
const stytchClient = useStytchB2BClient();
const { session } = useStytchMemberSession();
useEffect(() => {
if (session) {
window.location.href = 'https://example.com/profile';
} else {
const token = new URLSearchParams(window.location.search).get('token');
stytchClient.sso.authenticate({
sso_token: token
});
}
}, [stytchClient, session]);
return <div>Loading</div>;
Using the vanilla JS in HTML, this looks like:
<script>
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('PUBLIC_TOKEN');
const token = new URLSearchParams(window.location.search).get('token');
stytch.sso.authenticate({
sso_token: token
});
</script>
4Test it out
Run your application, and test out the flow using the Organization and SSO Connection you created earlier!