Backend Integration of MFA
This guide covers enrollment and authentication of MFA using an entirely backend approach, leveraging our backend SDKs or direct APIs.
The below sequence outlines the expected flow after primary authentication, when an Organization's MFA Policy is REQUIRED_FOR_ALL but the Member is not currently enrolled in MFA.
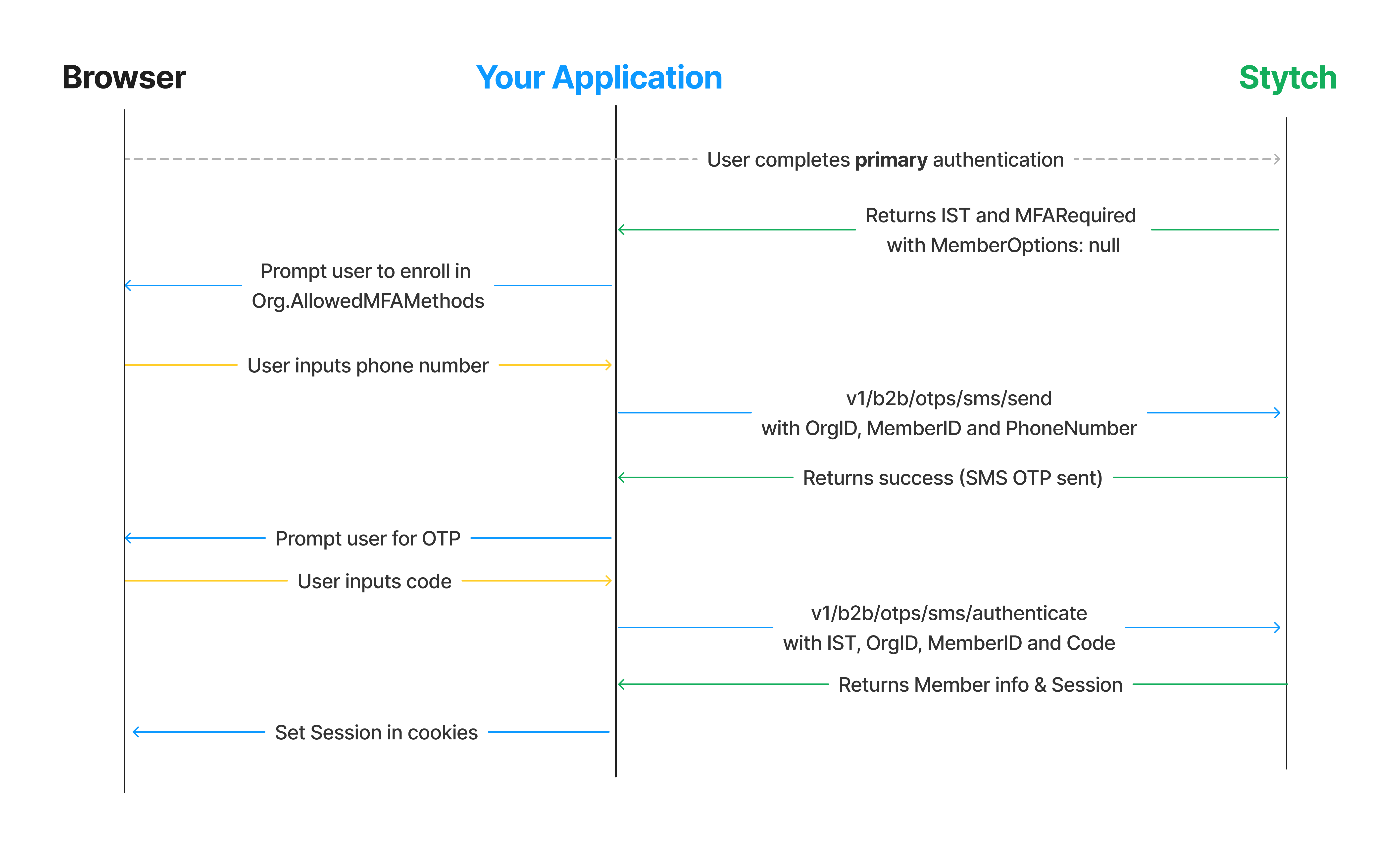
1Detect when MFA enrollment required
The following APIs will return a Member Session if the Member is not enrolled in MFA OR an intermediate_session_token and an mfa_required object that indicates MFA is required in order to be granted a Session for the Organization:
If the Member is not yet enrolled in MFA, the response will look as follows:
{
"intermediate_session_token": "oNJB3foIA79dn_uNVMNghG_MGkKSLHnR65NsKXv0gZzY",
"mfa_required": {
"member_options": null,
"secondary_auth_initiated": null
},
"member_authenticated": false,
"organization": {
"mfa_methods": "REQUIRED_FOR_ALL",
"allowed_mfa_methods": ["sms_otp", "totp"]
}
...
}
Indicating that member_authenticated: false due to mfa_required -- but no member_options are present.
For the primary authentication endpoint(s) you've already integrated add handling to detect when MFA enrollment is required by checking to see if the organization.mfa_methods is ALL_ALLOWED or RESTRICTED. If RESTRICTED use the organization.allowed_mfa_methods to surface only allowed MFA methods to the user.
def authenticate() -> str:
# Any primary auth flow, EML shown for demonstration
if request.args["stytch_token_type"] != "multi_tenant_magic_links":
return "unsupported auth method", 400
resp = stytch_client.magic_links.authenticate(magic_links_token=request.arg["token"])
if resp != 200:
return "something went wrong", 500
if resp.member_authenticated:
# MFA not required case, shown for demonstration
session['stytch_session'] = resp.session_token
return redirect(url_for('org_home', slug=resp.organization.organization_slug))
# Store IST to include in MFA authentication
session['ist'] = resp.intermediate_session_token
# Member must enroll in MFA
if resp.mfa_required.member_options is None:
# Only surface options that are allowed for the Organization
if resp.organization.mfa_methods == "ALL_ALLOWED:
sms_allowed = True
totp_allowed = True
else:
sms_allowed = ("sms_otp" in resp.organization.allowed_mfa_methods)
totp_allowed = ("totp" in resp.organization.allowed_mfa_methods)
return render_template(
'enrollMFA.html',
sms_allowed=sms_allowed,
totp_allowed=totp_allowed,
organization_id=resp.organization.organization_id,
member_id=resp.member.member_id
)
# Case where member already enrolled covered in next guide
return render_template(
'submitOTP.html',
organization_id=resp.organization.organization_id,
member_id=resp.member.member_id
)
Create a basic template that conditionally renders the MFA options to show to the user, and prompts them to enroll.
For TOTP no user input is required to start registration, but for SMS you'd want a form field for the user to input their phone number:
<form action="/enroll-sms" method="post">
<label for="phone">Phone Number:</label>
<input type="text" id="phone" name="phone" required>
<input type="hidden" name="organization_id" value="{{ organization_id }}">
<input type="hidden" name="member_id" value="{{ member_id }}">
<button type="submit">Submit</button>
</form>
2Start MFA enrollment
Add routes to handle starting the MFA enrollment process for SMS OTP or TOTP, depending on the users' selection.
@app.route("/enroll-sms", methods=["POST"])
def enroll_sms() -> str:
phone = request.form.get('phone', None)
organization_id = request.form.get('organization_id', None)
member_id = request.form.get('member_id', None)
if phone is None or organization_id is None or member_id is None:
return 'Missing required field', 400
# Make sure the `mfa_phone_number` is in the E.164 format, e.g. “+14155551234”.
resp = stytch_client.otps.sms.send(
organization_id=organization_id,
member_id=member_id,
mfa_phone_number=phone
)
if resp.status_code != 200:
return "Error sending SMS", 500
return render_template(
'inputMFACode.html',
organization_id=resp.organization.organization_id,
member_id=resp.member.member_id
)
@app.route("/enroll-totp", methods=["POST"])
def enroll_totp() -> str:
organization_id = request.form.get('organization_id', None)
member_id = request.form.get('member_id', None)
if organization_id is None or member_id is None:
return 'Missing required field', 400
resp = stytch_client.totps.create(
organization_id=organization_id,
member_id=member_id
)
if resp.status_code != 200:
return "Error sending EML", 500
return render_template(
'registerTOTP.html',
qr_code=resp.qr_code,
organization_id=resp.organization.organization_id,
member_id=resp.member.member_id
)
3Authenticate to complete enrollment
You'll want to add a template that allows the user to finish the enrollment process by authenticating with their chosen factor.
For SMS this would be a form that allows the user to input the OTP they received like:
<form action="/authenticate-mfa" method="post">
<label for="code">Input Code:</label>
<input type="code" id="code" name="code" required>
<input type="hidden" id="type" name="type" value="sms">
<input type="hidden" name="organization_id" value="{{ organization_id }}">
<input type="hidden" name="member_id" value="{{ member_id }}">
<button type="submit">Submit</button>
</form>
For TOTP, you'll need to first surface the QR Code so the user can create a TOTP registration in their authenticator app, and then input the TOTP code from that registration.
<body>
<h1>Scan QR Code and once registered, input TOTP code from your authenticator app:</h1>
<img src="{{qr_code}}" alt="QR Code">
<div>
<form action="/authenticate-mfa" method="post">
<label for="code">Input Code:</label>
<input type="code" id="code" name="code" required>
<input type="hidden" id="type" name="type" value="totp">
<button type="submit">Submit</button>
</form>
</div>
</body>
When the user submits their TOTP or OTP code, you'll call Stytch to authenticate the code. If successful, this will complete the Member's enrollment in MFA and grant them a Member Session.
@app.route("/authenticate-mfa", methods=["POST"])
def authenticate_mfa() -> str:
code = request.form.get('code', None)
organization_id = request.form.get('organization_id', None)
member_id = request.form.get('member_id', None)
mfa_type = request.form.get('type', None)
if code is None or organization_id is None or member_id is None:
return 'Missing required field', 400
ist = session.get('ist')
if not ist:
return 'No intermediate session token', 400
if mfa_type == 'sms':
resp = stytch_client.otps.sms.authenticate(
intermediate_session_token=ist,
code=code,
organization_id=organization_id,
member=member_id
)
elif mfa_type == 'totp':
resp = stytch_client.totps.authenticate(
intermediate_session_token=ist,
code=code,
organization_id=organization_id,
member=member_id
)
else:
return 'unsupported method', 400
if resp.status_code != 200:
return 'error authenticating mfa', 500
session.pop('ist', None)
session['stytch_session'] = resp.session_token
return redirect(url_for('org_home', slug=resp.organization.organization_slug))
The member is now enrolled in MFA, and will be prompted to perform MFA through their selected method on subsequent authentication.
4Test it out
Run your application and attempt to login to your Organization with the REQUIRED_FOR_ALL MFA Policy to test out required enrollment in MFA.