Allow apps to log in with your Stytch-powered app
In this guide, you'll learn how to configure your suite of OIDC applications to log in and perform actions on behalf of your users with your Stytch-powered app. This enables users of your Stytch app to use their in-house tools, external integrations, desktop apps, AI agents, any OIDC-compatible app to use your Stytch application as an Authorization Server, reducing user information duplication and simplifying the process of integrating Connected Apps with your app.
Fundamentals
OIDC is an industry standard for allowing applications to ask an Authorization Server—your Stytch-powered application—to verify the identity of a user. By logging in a user in this way we can consolidate information about a user’s authentication and authorization in Stytch and manage user data in one centralized location across multiple apps.
To ground our discussion about Connected Apps we will discuss the OIDC Authorization Code Flow (in the Connected App configuration, this is a "confidential app", described below). At the end of the article we will cover modification of this flow for "public apps" - Authorization Code Flow with PKCE.
The general flow for the Authorization Code Flow for a Connected App proceeds as follows:
- A user using the Connected App wishes to authenticate this app with your Stytch-powered Authorization Server in order to access user information (what we're implementing here). They click a button or follow a link to an Authorization URL (configured below) owned by your Stytch app.
- URL parameters provided to the Authorization URL page are caught by a Stytch component which proceeds with the login flow with Stytch.
- The user may be prompted to verify that the Connected App has permission to access their data (depending on whether the Connected App is "First Party" or "Third Party", described below)
- Upon success, Stytch redirects the browser to the configured redirect_uri owned by the Connected App with a code parameter.
- The Connected App uses this code parameter, along with its client_id and client_secret, to request an access_token (and optionally an id_token or refresh_token) from Stytch.
Once these steps are complete, the Connected App is logged in and can use the access_token to access user data.
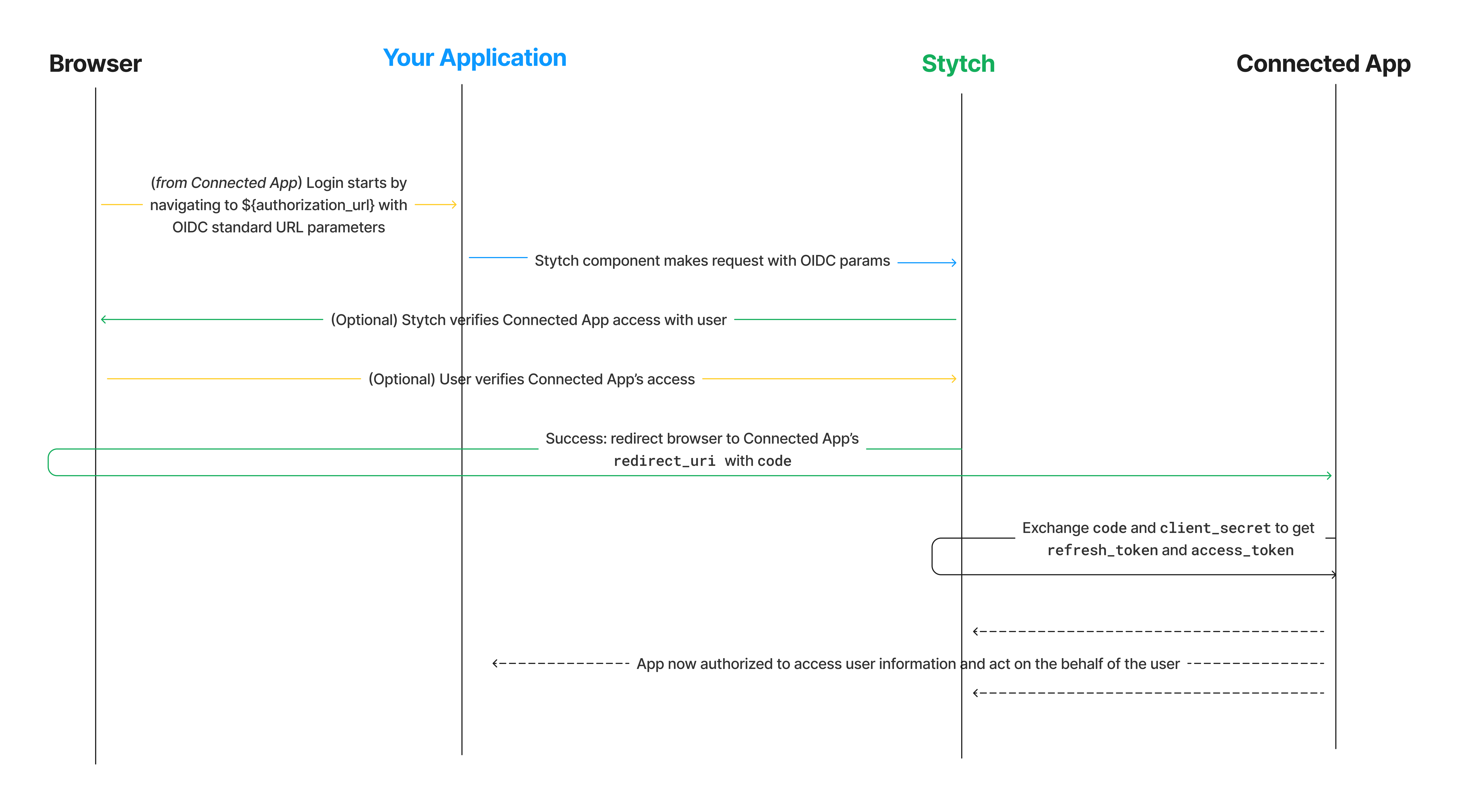
Before you start
In order to complete this guide, you'll need:
- A Stytch project. If you don't have one already, in the Dashboard, click on your existing project name in the top left corner of the Dashboard, click Create Project, and then select B2B Authentication or Consumer Authentication.
- A React or Next.js app authorized to use Stytch frontend SDKs
- A Relying Party app (the Connected App) that wishes to receive user information from your application.
Integration steps
1Implement the React component
In OIDC Authorization Code Flow, to begin the login flow the Connected App will be make a request to an Authorization URL in your Stytch-powered application. This page will host a Stytch component which assists in implementing the flow.
The redirect to this page should include these URL parameters as defined in the OIDC specification:
Name | Meaning |
---|---|
response_type | The response type of the OIDC request. For Authorization Code Flow this is always code |
scope | The scope of access of the user information. Typically one or more of openid profile email phone |
client_id | The Stytch client app id, which you will receive when configuring a Client App (below) |
redirect_uri | The URI owned by the Client App to call back when Stytch verifies access is granted. After the initial steps of the login process, Stytch redirects to this URI. You will configure this in Stytch when configuring a Client App (below) |
state (optional) | An opaque value (not used by the flow) which will be passed back and forth throughout the flow. This can be used in some enhanced security scenarios or to store information about user state that should be preserved throughout the flow. |
code_challenge | Required for public clients, see below |
code_challenge_method | Required for public clients, see below |
In order to use Stytch as an Identity Provider, we have created a component <B2BIdentityProvider /> for use in your app. When mounted on the page located at the Authorization URL, this component will catch the parameters passed in the URL and initiate the flow with Stytch. After the initial step succeeds, Stytch will respond with a redirect to send the user's browser to the redirect_uri with a code for the Connected App to complete the flow on its backend.
For this flow to succeed, the user must already be logged in to your Stytch-powered app and have an active session, so you'll want to check for a valid session prior to rendering the component and if the user is not logged in, redirect to the login page with a return_to parameter.
const { member } = useStytchMember();
if (!member) {
const loginURL = `/login?returnTo=${window.location.toString()}`
return <Redirect to={loginURL} />
}
return <B2BIdentityProvider />
2Configure Stytch for the component
We will configure your Stytch app in the Connected Apps section of the Stytch Dashboard. Enter the URL where the component is mounted into the Authorization URL field.
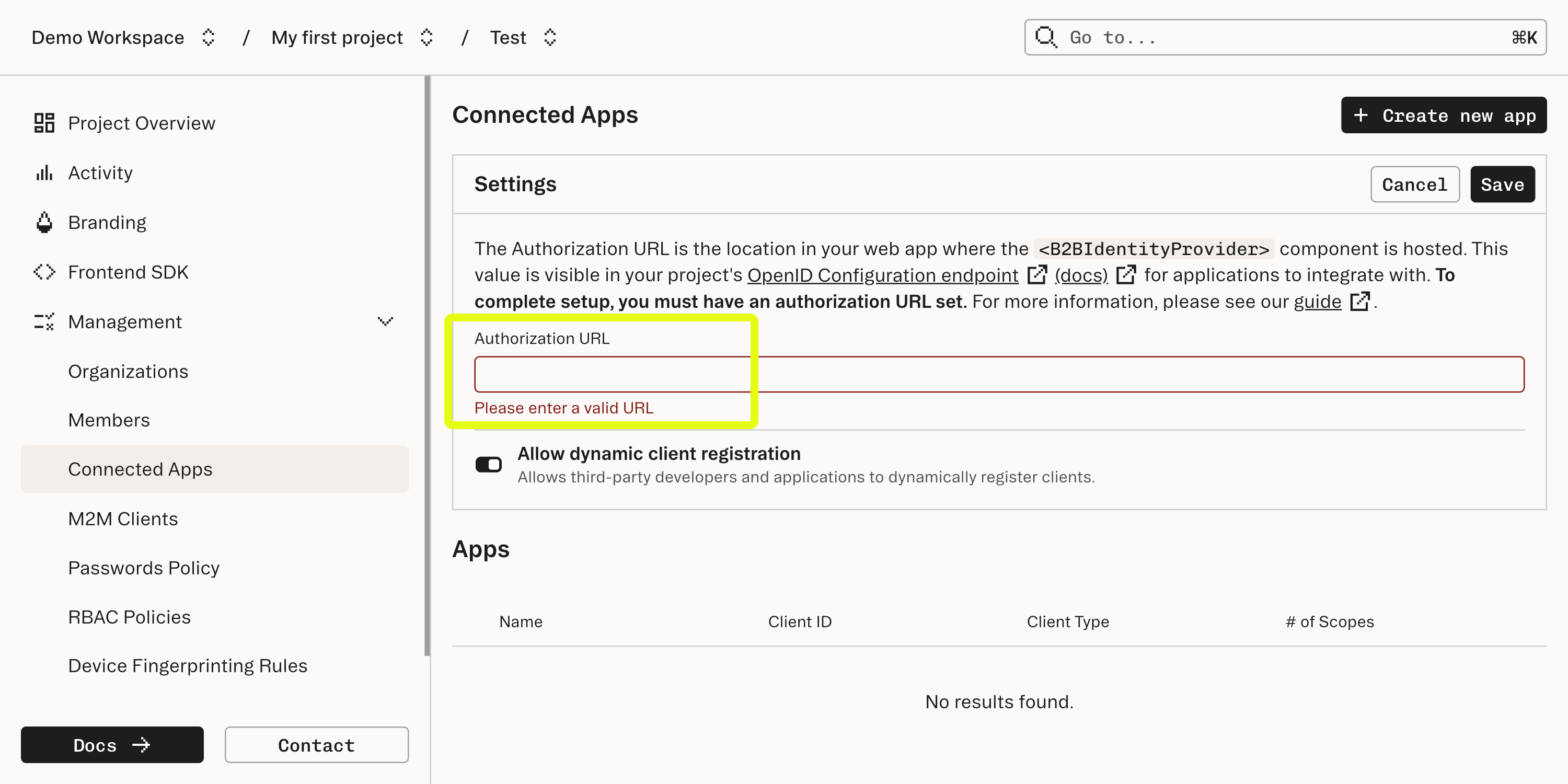
3Configure the Connected App(s) information
After Stytch handles verification of the user and the application’s ability to access the user’s information, Stytch will issue a redirect. This redirect is intended to go to a URL which should be supplied by the Connected App and provides some short-lived credentials that allow the Connected App to complete the authorization flow.
To set this up, each Connected App is also configured in the Connected Apps section of the Dashboard:
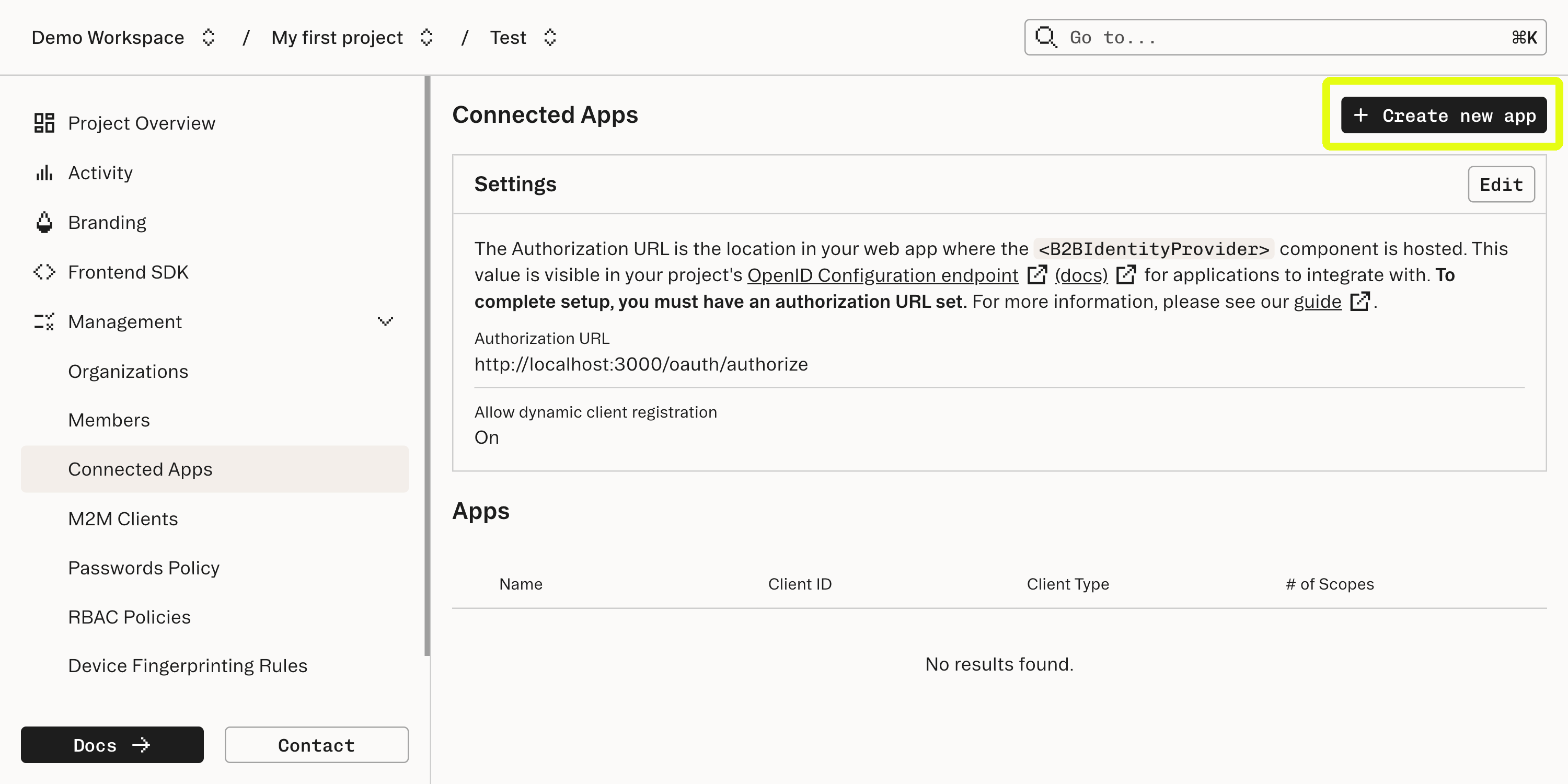
Upon creating a new Connected App we specify what type of user consent and OIDC flow the Connected App expects. Stytch supports connecting a few types of client apps which vary based on how much user consent is appropriate and specifics of the OIDC flow:
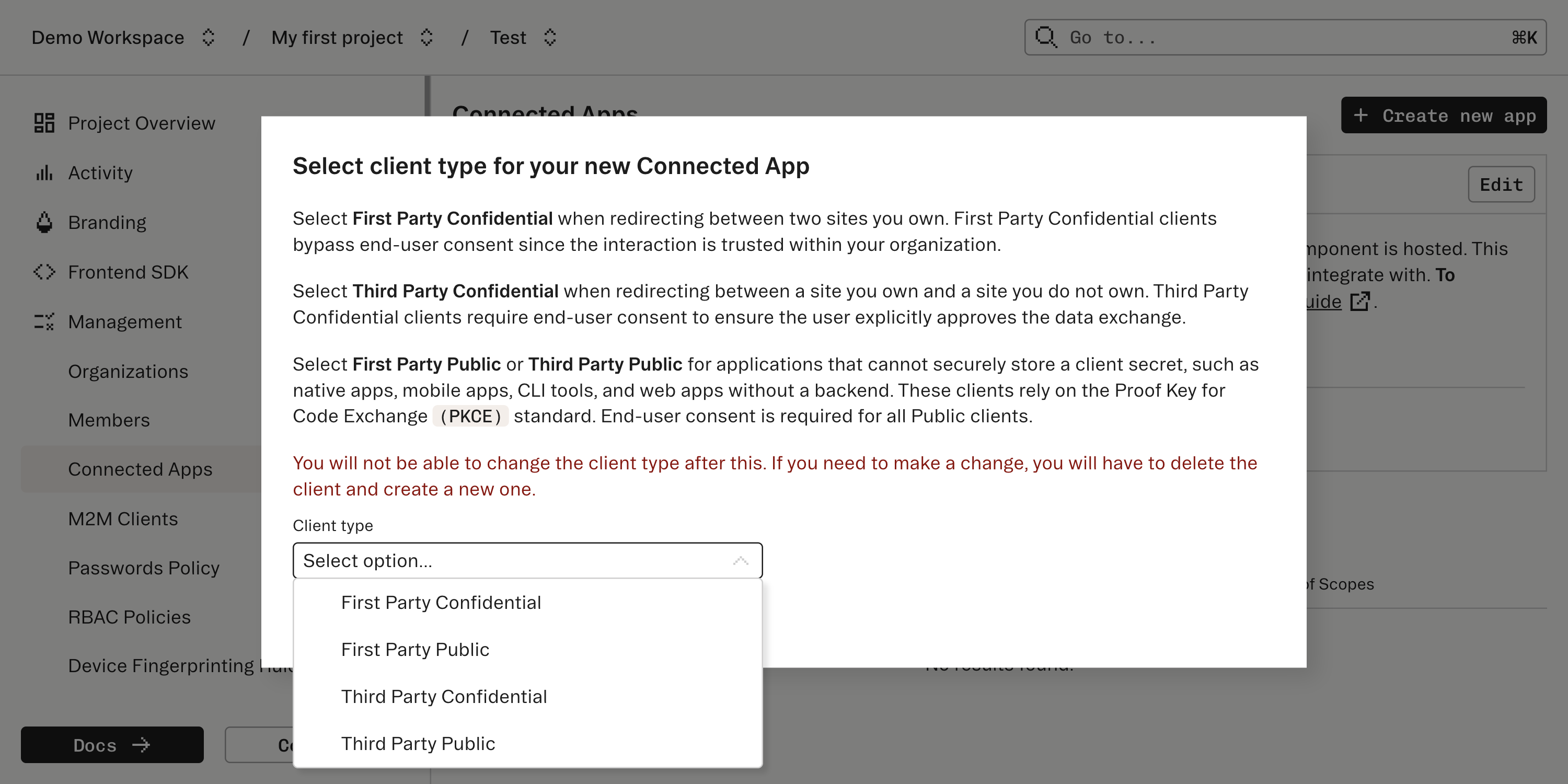
Continue to configure the Connected App on the next screen. Make sure to take a note of the “Client ID” and the “Client secret” (for confidential apps); these will be needed by the Connecting App to complete the Authorization Code Flow.
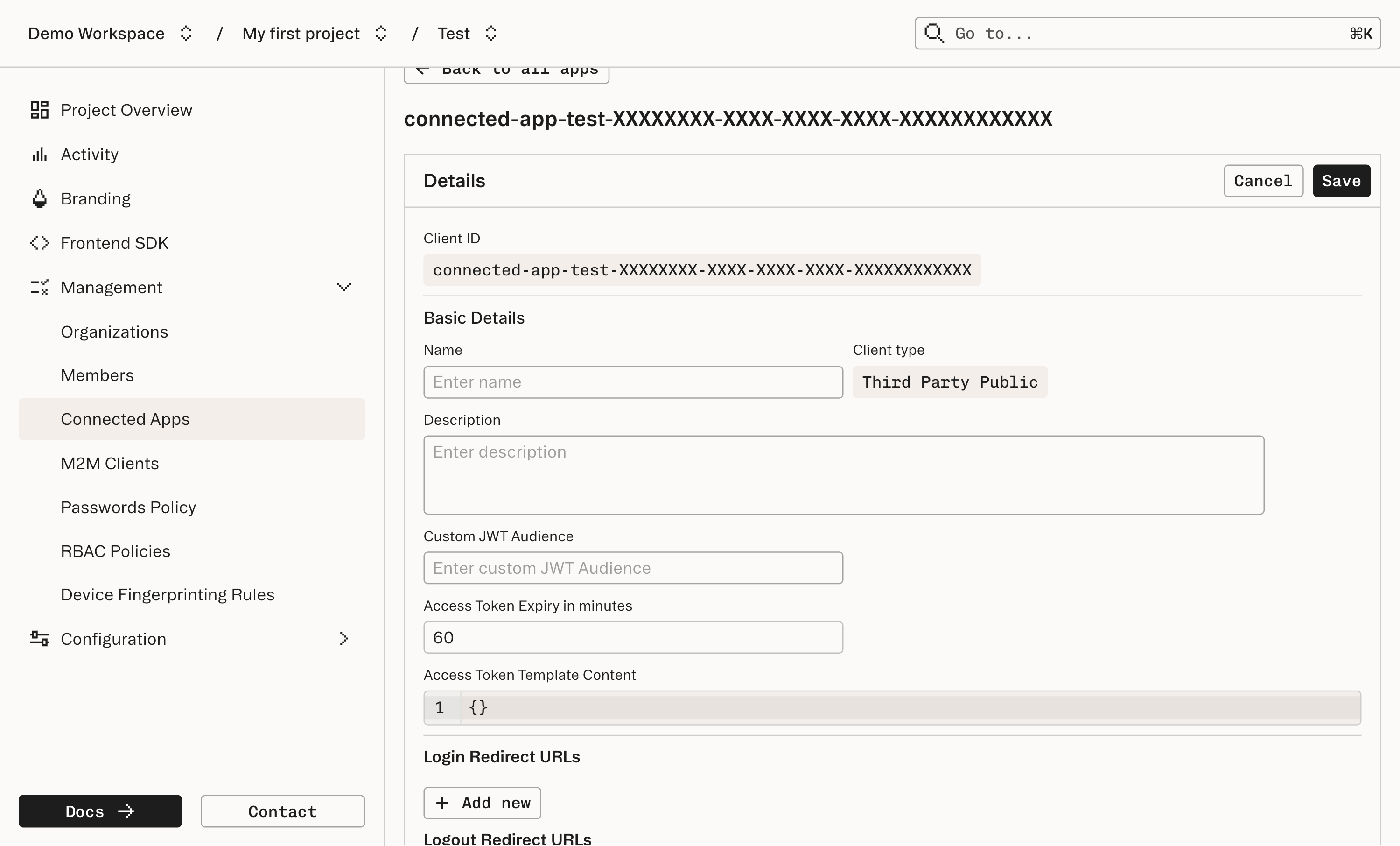
The Connected App will also supply a Redirect URL for receiving the login information from Stytch after the initial login is complete. This should be entered into the “Redirect URLs” field.
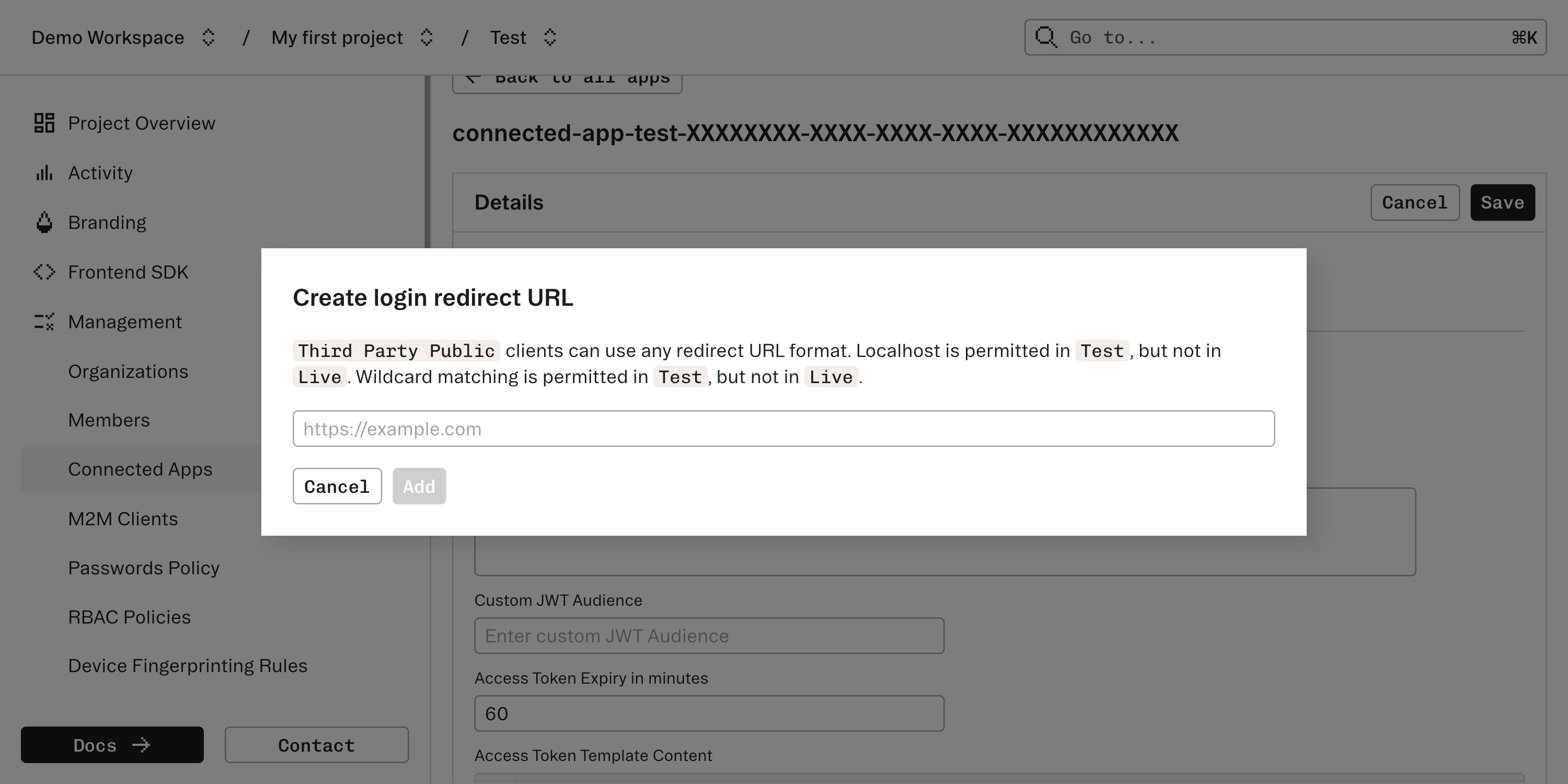
At this point, off-the-shelf software should be prepared to authorize as a Connected App with Stytch.
4Implementing the server side of the Authorization Code Flow
Generally off-the-shelf software will only need to be configured with the location of the Authorization URL, Client ID, and Client Secret and it should be prepared to authorize with Stytch and this section can be skipped. If, however, you are implementing your own Connected App, or just curious, it's worth understanding the second part of the Authorization Code Flow—what happens upon the Connected App receiving the redirect.
The server side flow is very similar to Stytch's M2M Authentication in that we will be exchanging the Connected App's own credentials for an access token. The difference in this case is that we have the code returned from the above steps. This token represents the ability of the client app to act on behalf of the user—in other words, conceptually the code can be thought of representing permission from the user to enable the app—which must also authorize itself in the request—to see their data and act on their behalf.
To perform the token exchange, just as in M2M Authentication, we will make a POST request to an endpoint in Stytch (or in a CNAMEd URL that points to Stytch). The endpoint is located at https://test.stytch.com/v1/public/$PROJECT_ID/oauth2/token (or similar, depending on your Stytch test or production environment or CNAME settings).
The request should contain these parameters:
Name | Meaning |
---|---|
grant_type | The type of auth grant we're seeking. For Authorization Code Flow this is always authorization_code |
code | The code received in a parameter of the request sent to the Redirect URL |
client_id | The Stytch client app id, which you will receive when configuring a Client App (below) |
client_secret | The Stytch Connected App's Client Secret - required for confidential clients |
redirect_uri | While there is no redirect issued from this request redirect_uri must be present and identical to the value used in the first part of the flow |
code_verifier | Required for public clients, see below |
For example, here is what such a request might look like using curl:
curl --request POST \
--url https://test.stytch.com/v1/public/$PROJECT_ID/oauth2/token \
-d grant_type="authorization_code" \
-d client_id="$CONNECTED_APP_CLIENT_ID" \
-d client_secret="$CONNECTED_APP_CLIENT_SECRET" \
-d code="$CODE_FROM_REDIRECT_PARAMETERS" \
-d redirect_uri="$REDIRECT_URL"
The response from this request will be a JSON payload with the access token for authorizing requests from the Client App.
Public apps: Authorization Code Flow with PKCE
A potential vulnerability exists in the OIDC flow due to the nature of it requiring two asynchronous requests to complete. A malicious party could intercept the code returned in the first step of authorization and attempt to use it to authorize their own app before the legitimate app exchanges it for an access_token. In the case of the Authorization Code Flow this is prevented by the fact that the pre-shared Client Secret is not known to the malicious actor so they don't have enough information to do the complete code exchange.
Due to the nature of, for instance, in-browser single-page apps with no backend, or mobile apps where secrets can be extracted from the app if distributed within the app, there is sometimes no location to safely store the client_secret. We call these "Public" apps. For these apps, we implement a variant of the Authorization Code Flow using PKCE (Proof Key for Code Exchange). This flow uses an extension to the Authorization Code Flow to ensure both requests used during the Authorization Code Flow come from the same Connected App.
When beginning the authorization request, the Connected App will construct a code_verifier (for example, generating a cryptographically random value) and use it to generate a code_challenge. The code_challenge is the SHA-256 hash of the code_verifier. On the initial request to Stytch—the request made to the page hosting the <IdentityProvider \> component—the additional URL parameters of code_challenge and code_challenge_method should be sent:
Name | Meaning |
---|---|
code_challenge | The SHA-256 hash of the value of the code_verifier |
code_challenge_method | The method of hashing the code_verifier. For Stytch this should always be S256 |
Upon receipt of this request, Stytch will save the value of code_challenge for later. After the user has granted permission for access, Stytch will return a code from this step just as in the regular Authorization Code Flow.
During the code exchange part of the process, the Public app will exchange the code for an access_token as the server would do in the Authorization Code Flow. However, the Public app has no access to a client_secret so cannot send that parameter. Instead, the Public app also sends the original code_verifier with this request:
Name | Meaning |
---|---|
code_verifier | The original value used to generate the code_challenge |
Stytch will verify that its saved code_challenge was generated from this code_verifier before generating and returning credentials for the app. In this way it can be sure that the app that requested the code in the first request is the same app that is requesting the access_token in the second request.
OIDC Compliance and the issuer field
To ensure your Stytch-powered application is fully OpenID Connect (OIDC) compliant, the issuer field in your JWTs must be an https URL that matches the domain of your identity provider.
How Stytch determines the issuer:
- If you use a custom domain (CNAME):
- When your users authenticate via a custom domain (e.g., https://login.yourcompany.com), Stytch will set the issuer in your JWTs to your custom domain.
- This is fully OIDC compliant and required by many OIDC clients and libraries.
- If you do not use a custom domain:
- The issuer will default to stytch.com/$project_id.
- These formats are not fully OIDC compliant and may cause compatibility issues with OIDC clients that strictly enforce the spec.
To get a fully OIDC-compliant issuer:
- Set up a custom domain (CNAME) in your DNS that points to Stytch and configure Stytch to recognize this domain.
- Configure your application to make all API calls to Stytch over this domain.
- Use this domain for all authentication flows.
Why does this matter?
- Many OIDC clients and libraries will reject tokens if the issuer does not match the expected https URL.
- Using a custom domain ensures maximum compatibility and security for your integrations.
Note: If you are using Stytch's default domain and encounter issues with OIDC integrations, setting up a custom domain is the recommended solution.
What's next
Read more about our other offerings: