Stytch and frontend development (headless)
Our frontend SDKs can be utilized headlessly for developing and managing your auth client-side. With a robust set of headless features, our frontend SDKs not only allow you to create custom UIs and screens but also provide you with controls to customize auth flows. This guide covers the fundamental aspects and key considerations when implementing our frontend SDKs headlessly.
Here’s a list of libraries and options we offer for frontend development that can be used headlessly:
- Frontend SDKs
- Mobile SDKs
Refer to the SDK reference page for full documentation. You can also explore the example apps to get hands-on with the code.
Overview
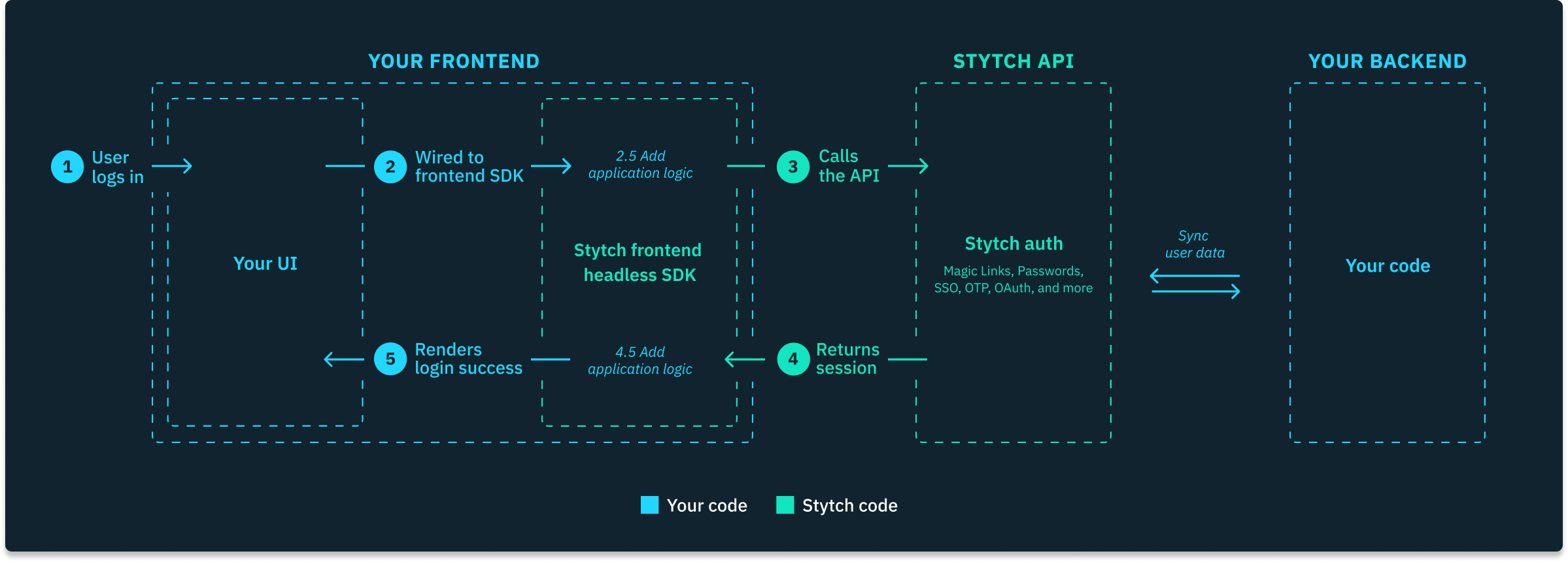
At a high-level, implementing Stytch headlessly on the client-side involves the following steps:
- The end user attempts to log into your application with your custom-built UI.
- Your frontend handles the UI events, collects all the necessary authentication data, and then utilizes Stytch’s frontend SDK methods to call the Stytch API to perform an auth-related operation.
- Stytch API processes the request and returns a response with pertinent data.
- Your frontend handles the response as needed, which may involve calling the Stytch API again, updating your UI, or relaying the data to your backend.
- Once the end user successfully authenticates, Stytch’s frontend SDK automatically manages the storage of session tokens using browser cookies or mobile storage.
Using the frontend SDK headlessly enables you to build your own custom UI, customize auth flows, and handle user and session management — directly on the frontend. Our frontend SDK supports most features of the Stytch platform; however, there are certain operations that can only be done with a backend implementation.
Code examples
Here are some example code snippets that demonstrate the general idea of implementing Stytch headlessly.
Authentication flows
// Start the authentication flow
import { useStytch, useStytchUser, useStytchSession } from '@stytch/nextjs';
const Login = () => {
const stytchClient = useStytch();
const sendEmailMagicLink = async () => {
await stytchClient.magicLinks.email.send('sandbox@stytch.com', {
login_magic_link_url: "http://localhost:3000/authenticate",
login_expiration_minutes: 5,
});
};
return <button onClick={sendEmailMagicLink}>Login</button>;
};
...
// Complete the authentication flow
const AuthenticateEML = () => {
const stytchClient = useStytch();
const router = useRouter();
useEffect(() => {
const token = router?.query?.token?.toString();
stytchClient.magicLinks.authenticate(token, {
session_duration_minutes: 60,
});
}, [router, stytchClient]);
...
Session management
// Validate session for protected pages and resources
const isAuthenticated = () => {
const { session, fromCache } = useStytchSession();
const router = useRouter();
useEffect(() => {
if (session && !fromCache) {
// there's an active session
router.replace("/profile");
}
}, [session, fromCache, router]);
...
// Revoke session
const LogOut = () => {
const stytchClient = useStytch();
const revokeSession = async () => {
await stytchClient.session.revoke();
router.push("/");
};
return <button onClick={revokeSession}>Log out</button>
...
User management
// Manage user records
const UpdateProfile = (user) => {
const stytchClient = useStytch();
const updateUser = async (e) => {
e.preventDefault();
const data = new FormData(e.target);
await stytchClient.user.update({
name: {
first_name: data.get('first-name'),
last_name: data.get('last-name'),
},
untrusted_metadata: {
profile_pic_url: data.get('profile-pic-url'),
}
});
};
return <form onSubmit={updateUser}>...</form>
...
Considerations when using our frontend SDKs
Session token storage
Our frontend JavaScript SDK automatically stores all minted Stytch session_token and session_jwt values in browser cookies or mobile storage. To strike the best balance between developer experience and security, by default, these cookies are not HttpOnly. If HttpOnly cookies are a requirement for you, you can enable them following our documentation. Alternatively, you can use a backend-only Stytch integration, where you can fully customize where and how session tokens are stored.
You can also allow session cookies to be available to all subdomains, e.g. api.yourapp.com, by configuring the StytchClientOptions. See this documentation for all options for cookie settings.
Hydrating sessions on the backend
Session authentication, specifically after the initial user login, should be implemented on the backend in order to protect your application’s sensitive routes, data, or actions by authenticating each request’s session token or JWT.
If you’re authenticating users and minting sessions with our frontend SDKs, browser cookies will automatically be created for the session. As long as your backend shares a domain with your frontend, the session_token and session_jwt cookies will be included in the request headers on every call made to your backend. Your application can then use Stytch’s backend API endpoints to authenticate the session before returning any protected resources to your frontend.
You can read more about session hydration here.
MFA requirements
For security purposes, our frontend SDK requires users to complete MFA (multi-factor authentication) before they can take certain sensitive user management actions. You can read more about this in our Multi-factor authentication SDK resource. When using a backend-only Stytch integration, you can fully control when to require MFA.
Backend-restricted operations
Certain Stytch operations and features are only available as backend implementations. These include but are not limited to:
- Setting custom claims on sessions
- Deleting Stytch Users
- Searching for Stytch Users
- Updating a User’s trusted_metadata
PKCE (Proof Key for Code Exchange)
PKCE (Proof Key for Code Exchange) is a specification that makes certain login flows more secure by cryptographically validating that the flow starts and ends on the same device. When using our frontend JavaScript SDK, you can turn PKCE on or off for each Stytch product by simply flipping a toggle in the Frontend SDKs tab in the Stytch Dashboard.
For security purposes, PKCE is required when using any of our frontend mobile SDKs and when using a deep link as a redirect URL. When using a backend-only Stytch integration, you will be required to complete some additional implementation steps to enable PKCE, if desired.
Account enumeration
Some of our frontend methods do not fully protect against account enumeration by design. To create the optimal UX, our frontend SDK sometimes returns errors that indicate whether or not an account associated with a given email address or phone number exists.
If account enumeration is a concern for your application, we recommend taking one of the following approaches:
- Turn off certain frontend functionality like the Passwords product and the Send flow for OTP and Email Magic Links, which can be toggled via the Frontend SDKs tab in the Stytch Dashboard.
- Enable opaque errors, which can be found under optional configuration on the Frontend SDKs tab. Toggling this feature transforms any errors returning by the frontend SDK that reveal whether a given email or phone number is associated with an account. Additionally, turning on this feature prevents the Send methods from returning an error if a phone number or email is not found and disables the Passwords product entirely.
- Use a backend-only Stytch integration.
Toll fraud
When our SMS OTP or WhatsApp OTP products are enabled for your project via the Frontend SDKs tab in the Stytch Dashboard, anyone with access to your Stytch public_token can trigger SMS or WhatsApp messages to be sent on your project’s behalf.
Unless you plan to use our Device Fingerprinting Protected Auth feature, you may be vulnerable to toll fraud attacks – particularly if you’re interested in enabling SMS to phone numbers outside of the US and Canada.
See our toll fraud resource for additional information.
What’s next
Read our backend implementation guide.
If you have additional questions about our different integration options, please feel free to reach out to us in our community Slack, our developer forum, or at support@stytch.com for further guidance.