This is an integration guide for setting up the Stytch SDK in your app. First, you'll configure our frontend SDK in your client code to create a login experience in your app. Then, you'll add support to your server code for authenticating Stytch magic links. If you have any questions, check out the docs for the SDK or our example app that uses the code in this guide.
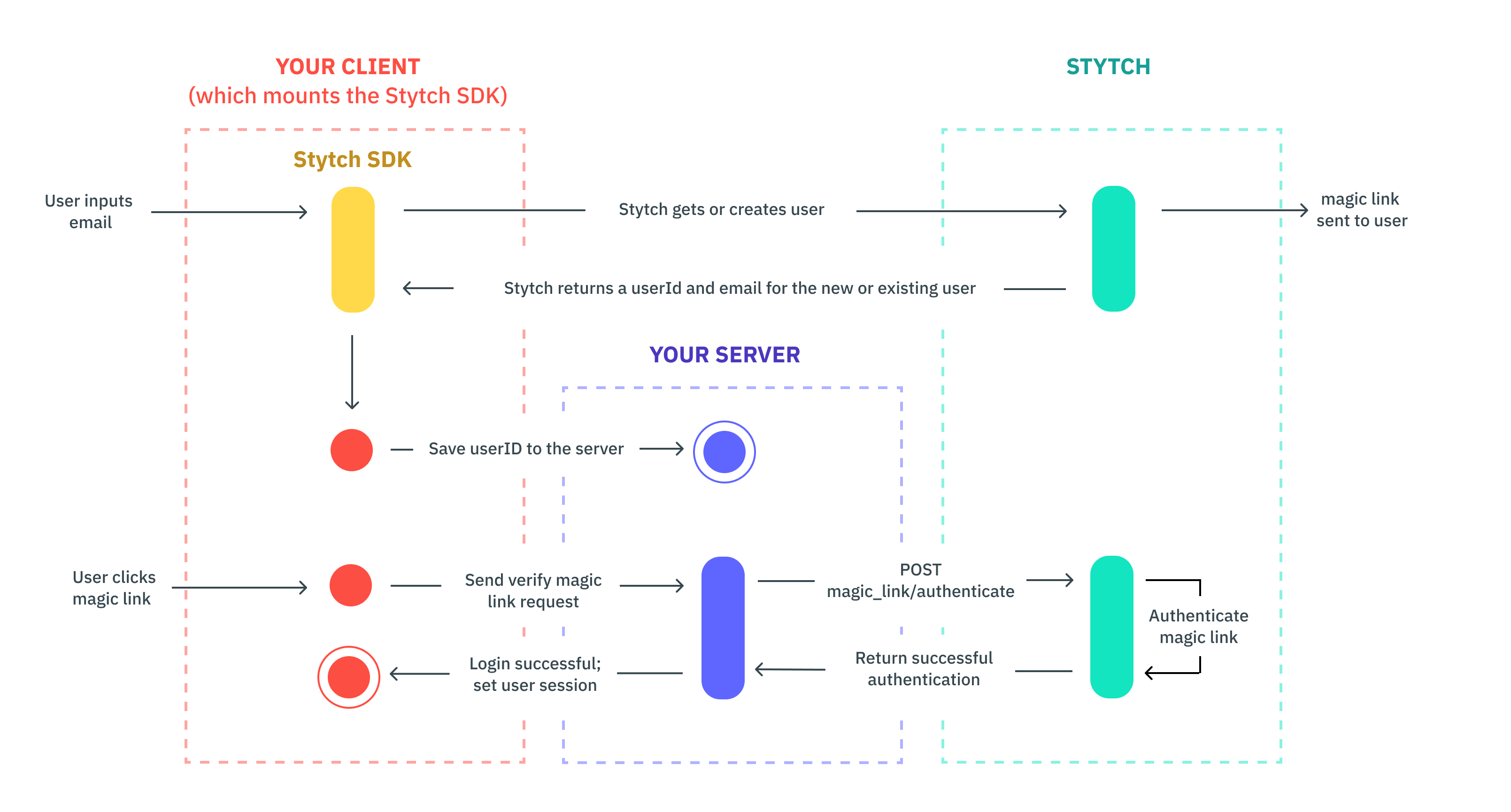
Step 1: Configure your project in the Stytch dashboard
Before adding Email magic links to your application you will need to make the following project configurations within the Stytch dashboard:
Create a Login and Sign-up redirect URL on the redirect URL configuration page.
Within SDK Configuration, authorize the domain(s) (eg. http://localhost:3000) the SDK will run on. Under Auth methods enable the Email magic links > Enable the LoginOrCreate Flow toggle.
Finally, under API keys, save your project's public_token for later.
Step 2: Use Stytch
2.1 Install Stytch
Install the @stytch/vanilla-js packages via npm or yarn. The packages provides frontend support for the Javascript SDK.
npm install @stytch/vanilla-js --save
2.2 Create Stytch Client
Initialize the Stytch Client
<script>
import { StytchUIClient } from '@stytch/vanilla-js';
const stytch = new StytchUIClient('PUBLIC_TOKEN');
</script>
<div id="stytch-login-ui"></div>
2.3 Configure your Login Component
Create a config object to initalize the magic link product in the JavaScript SDK.
<script>
import { StytchUIClient, Products } from '@stytch/vanilla-js';
const stytch = new StytchUIClient('PUBLIC_TOKEN');
const config = {
emailMagicLinksOptions: {
loginExpirationMinutes: 30,
loginRedirectURL: 'https://example.com/authenticate',
signupExpirationMinutes: 30,
signupRedirectURL: 'https://example.com/authenticate',
},
products: [
Products.emailMagicLinks,
],
};
stytch.mountLogin({ elementId: "#stytch-login-ui", config })
</script>
<div id="stytch-login-ui"></div>
2.4 Customize your Login Component
Customize the style of the SDK. The style object allows you to modify the look and feel to match your app.here.
<script>
import { StytchUIClient, Products } from '@stytch/vanilla-js';
const stytch = new StytchUIClient('PUBLIC_TOKEN');
const config = {
emailMagicLinksOptions: {
loginExpirationMinutes: 30,
loginRedirectURL: 'https://example.com/authenticate',
signupExpirationMinutes: 30,
signupRedirectURL: 'https://example.com/authenticate',
},
products: [
Products.emailMagicLinks,
],
};
const styles = {
fontFamily: '"Helvetica New", Helvetica, sans-serif',
};
stytch.mountLogin({ elementId: "#stytch-login-ui", styles, config })
</script>
<div id="stytch-login-ui"></div>
Step 3: Add server side support to your app for Stytch
Stytch works best with support from your app's server. You'll need to create or modify a few endpoints.
The Node examples use express to create http endpoints and express-session for sessions, but they can be replaced with any other http framework.
3.1 Install Stytch
Install the stytch package via npm or yarn. The stytch package provides backend support for the Stytch API.
npm install stytch --save
3.2 Create a user
POST/PUT user endpoint: When a user logs in to your app for the first time, the SDK will create a Stytch user for them and return a userId. We recommend adding a stytchUserId field to your user to save it.
app.post('/users', function (req, res) {
var stytchUserId = req.body.userId;
// TODO: Save stytchUserId with your user record in your app's storage
res.send(`Created user with stytchUserId: ${stytchUserId}`);
});
3.3 Authenticate a user: GET route
GET authentication endpoint: When a user enters their email and clicks the login button, we will send them an email with a magic link to log in. You need to create a route for the magic link. There are a few ways to do this:
- Implement a GET route on your frontend that accepts a token and passes it to a route on your backend that passes the token to Stytch to authenticate. This is recommended if your frontend lives separately from your backend.
- Implement a GET route in your backend that accepts a token and passes it to Stytch to authenticate. This is recommended if your backend and frontend live in the same service.
3.4 Authenticate a user: POST route
POST to Stytch: If your url in the previous step was https://example.com, your user will be directed to https://example.com?token={token} when they click the magic link in the email. You'll pass this token to Stytch to authenticate it. If authentication is successful, create a user session and log them into your app. If you'd like to keep this user logged-in for a while, include "session_duration_minutes": 60 (an hour, for example). Check out the session management guide to learn how to handle the session.
const stytch = require('stytch');
const client = new stytch.Client({
project_id: "PROJECT_ID",
secret: "SECRET",
env: stytch.envs.test
});
app.get('/authenticate', function (req, res) {
var token = req.query.token;
client.magicLinks.authenticate(token)
.then(response => {
req.session.authenticated = true;
req.session.save(function(err) {
console.log(err);
});
res.redirect('/home')
})
.catch(error => {
console.log(error);
res.send('There was an error authenticating the user.');
});
});