Create a CLI app with Stytch Authentication
In this guide, we'll walk through the creation of a simple Command Line Interface application which utilizes Stytch Connected Apps for authentication via an existing web application.
This guide will reference two example apps:
- stytch-react-example - used as the base web app that will authorize the CLI app.
- stytch-connected-apps-cli-example - a basic CLI app made with Cobra.
Feel free to pull down both of these example repositories to follow along and run through this guide!
Users will initiate authorization from within the CLI, redirect to the web app, authorize the CLI app from within the web app, and then be redirected back to the CLI where a Connected Apps access_token will be minted.
Before you start
In order to complete this guide, you'll need:
- A Stytch project. In the Stytch Dashboard, click on your existing project name at the top of the Dashboard, click "Create project", and select "Consumer Authentication".
- A web app that uses Stytch for authentication (this guide will use stytch-react-example).
- A basic CLI app (this guide will use stytch-connected-apps-cli-example).
1Create a Connected App
Navigate to the Connected Apps section of your Stytch Dashboard, and create a new Connected App.
Configure the app as First Party Public:
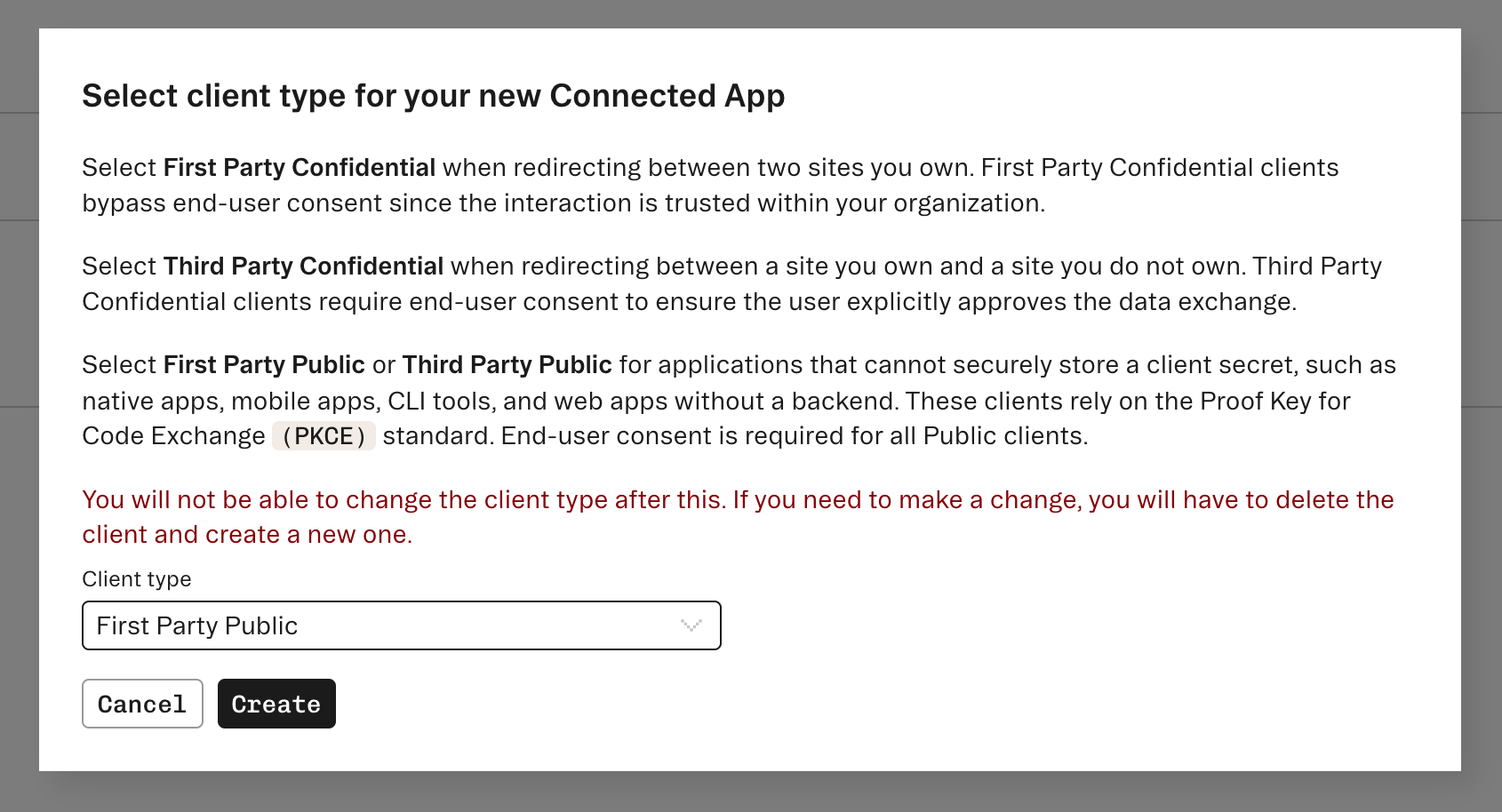
Add http://127.0.0.1/callback as a login redirect URL in the Connected App configuration - this is the URL our CLI app will use to receive the authorization code.
Omitting a port in the redirect URL permits any port to be used, allowing the CLI application to use dynamic port allocation. We want to dynamically allocate a free port, as opposed to picking a static one, as we don’t know what conflicting processes may be running on our end users device.
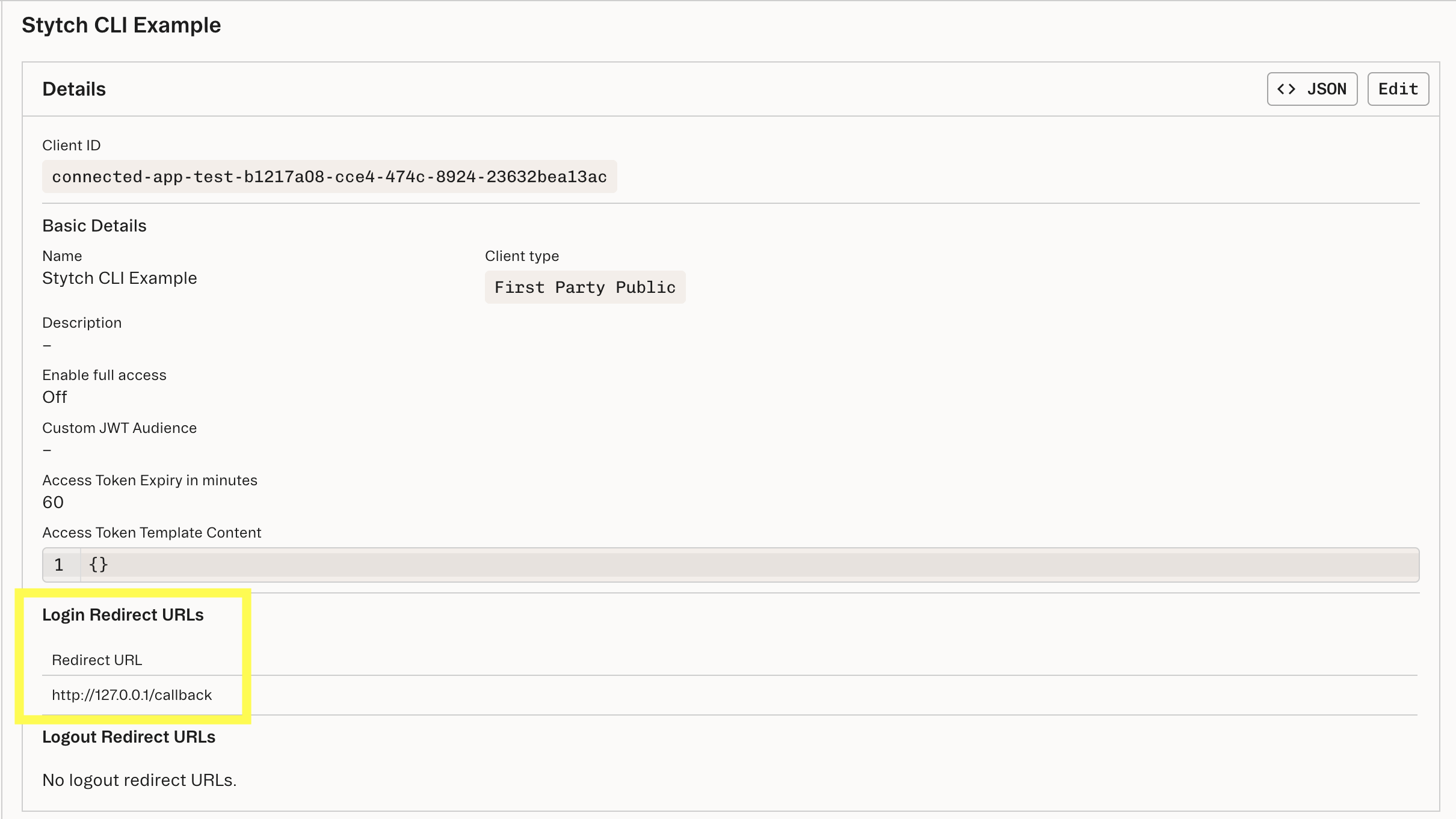
Set the authorization URL in Connected Apps tab to http://localhost:3000/oauth/authorize - this is the URL that our web app will expose to mount the <IdentityProvider /> component for authorization.
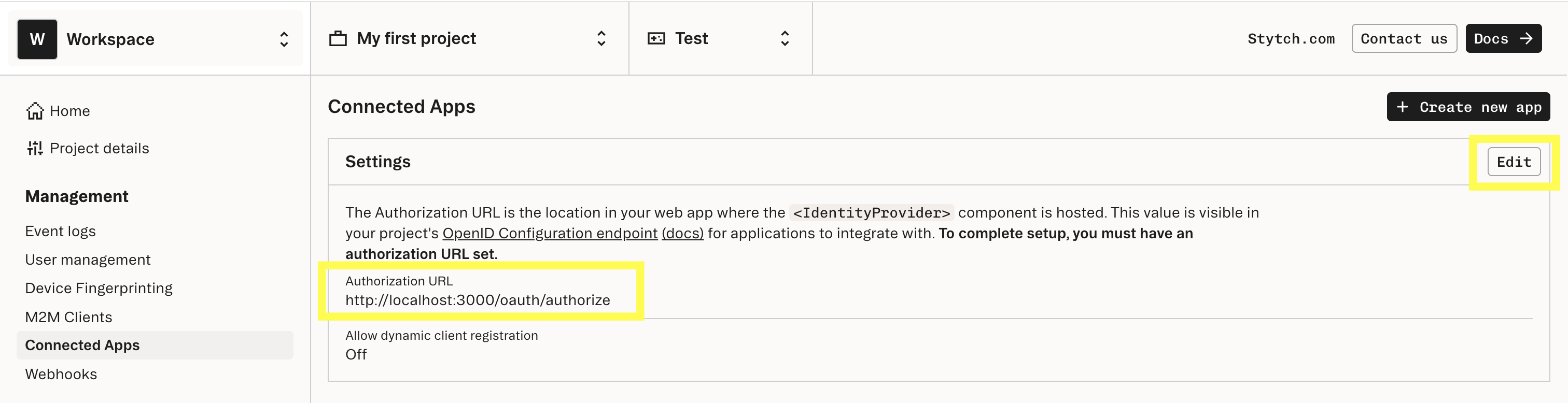
2Add an authorization route to your web app
Add a route in your web app that mounts the <IdentityProvider /> component.
In stytch-react-example, we do this here:
import { IdentityProvider, useStytchUser } from '@stytch/react';
import { useEffect } from 'react';
const Authorize = () => {
const { user } = useStytchUser();
useEffect(() => {
if (!user) {
window.location.href = '/';
}
}, [user]);
return <IdentityProvider />;
};
export default Authorize;
3Configure CLI app to initiate authorization flow with PKCE
To initiate the authorization flow, configure your CLI app to navigate to your web app’s page that mounts <IdentityProvider />.
The list of URL parameters required for this request can be found here.
If you’d like to receive a refresh_token, allowing your CLI app to refresh the access_token without the user re-authorizing, make sure your request includes scope=offline_access.
Because this is a public Connected App, we need to use PKCE rather than a client_secret, as there is nowhere to securely store a client_secret. The PKCE code_challenge and code_challenge_method will be sent along as part of the initiating request.
In stytch-connected-apps-cli-example, we do this in cmd/auth.go.
Relevant snippets below:
// Get a free port for the callback server
port := utils.GetOpenPort()
redirectURI := fmt.Sprintf("http://127.0.0.1:%d/callback", port)
// Generate PKCE values
codeVerifier, err := generateCodeVerifier()
if err != nil {
fmt.Printf("Error generating code verifier: %v\n", err)
return
}
codeChallenge := generateCodeChallenge(codeVerifier)
// Construct the auth URL with PKCE parameters
params := url.Values{}
params.Add("client_id", clientID)
params.Add("redirect_uri", redirectURI)
params.Add("response_type", "code")
params.Add("code_challenge", codeChallenge)
params.Add("code_challenge_method", "S256")
params.Add("scope", "offline_access")
authURL := fmt.Sprintf("%s?%s", authorizeURL, params.Encode())
fmt.Println("Opening browser for authentication...")
// Open the browser with the auth URL
err = openBrowser(authURL)
if err != nil {
fmt.Println("Please open the following URL in your browser:", authURL)
}
4Handle callback and exchange code for access token
Next, set up a callback handler in your CLI app that authenticates the authorization code received back from <IdentityProvider /> via the Get Access Token endpoint.
Note that we need to include code_verifier in this call since this is a Public app.
In stytch-connected-apps-cli-example, we do this in cmd/auth.go:
// Channel to receive the auth code
codeChan := make(chan string)
errorChan := make(chan error)
// Set up the callback handler
http.HandleFunc("/callback", func(w http.ResponseWriter, r *http.Request) {
code := r.URL.Query().Get("code")
if code == "" {
errorChan <- fmt.Errorf("no code received in callback")
return
}
// Send success response to browser
w.Write([]byte("Authentication successful! You can close this window."))
codeChan <- code
})
// Start the server in a goroutine
go func() {
if err := server.ListenAndServe(); err != http.ErrServerClosed {
errorChan <- err
}
}()
// Wait for either the code or an error
var code string
select {
case code = <-codeChan:
fmt.Println("Received authorization code")
case err := <-errorChan:
fmt.Printf("Error: %v\n", err)
return
case <-time.After(5 * time.Minute):
fmt.Println("Timeout waiting for authentication")
return
}
// Exchange the code for a token
token, err := exchangeCodeForToken(code, codeVerifier)
// Save the tokens to keyring
utils.SaveToken(token.AccessToken, utils.AccessToken)
utils.SaveToken(token.RefreshToken, utils.RefreshToken)
// Shutdown the server
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
server.Shutdown(ctx)
exchangeCodeForToken() in the above snippet is implemented here, and calls the Get Access Token endpoint with:
- The code retrieved by the channel set up,
- The code_verifier generated at the start of the authorization flow
- The Connected App’s client_id
- `grant_type: “authorization_code” because this is an initial authorization flow and not a refresh flow.
The access and refresh tokens returned should be stored securely, where other CLI applications or tools cannot access them. The example above uses a helper called utils.saveToken, which utilizes go keyring.
// SaveToken persists the token securely
func SaveToken(tok string, typ TokenType) error {
return keyring.Set(service, user+string(typ), tok)
}
5Testing
To test the flow with the example apps above:
- Set up and run stytch-react-example locally, following the instructions in the README.
- Authenticate into the example app, so that you have an active Session.
- Run stytch-connected-apps-cli-example, following the instructions in the README.
- You should be automatically redirected to the authorization flow in your browser, redirect back to your CLI, and see a Connected Apps access_token!
6Next steps
Now that you’re authenticated with a Connected Apps access_token in your CLI app, your backend can authenticate API requests by verifying this token.
For example, if your CLI app calls GET https://yourapp.com/api/resources to take some action in your app/platform, attaching the access_token as a Bearer token, your backend can perform authentication via something like the following:
func resourcesHandler(w http.ResponseWriter, r *http.Request) {
auth := r.Header.Get("Authorization")
if !strings.HasPrefix(auth, "Bearer ") {
http.Error(w, "Unauthorized", http.StatusUnauthorized)
return
}
token := strings.TrimPrefix(auth, "Bearer ")
resp, err := stytchClient.IDP.IntrospectTokenLocal(r.Context(), &idp.IntrospectTokenLocalParams{
Token: token,
})
if err != nil || !resp.TokenActive {
http.Error(w, "Unauthorized", http.StatusUnauthorized)
return
}
// Token is valid — respond with protected data
w.WriteHeader(http.StatusOK)
w.Write([]byte(`{"message": "Authenticated!"}`))
}